Setting and Using Cookies in React
- Category
- Guides
- Published
Learn how to set up cookies in React with this guide! You'll create a login page and store user information using cookies.
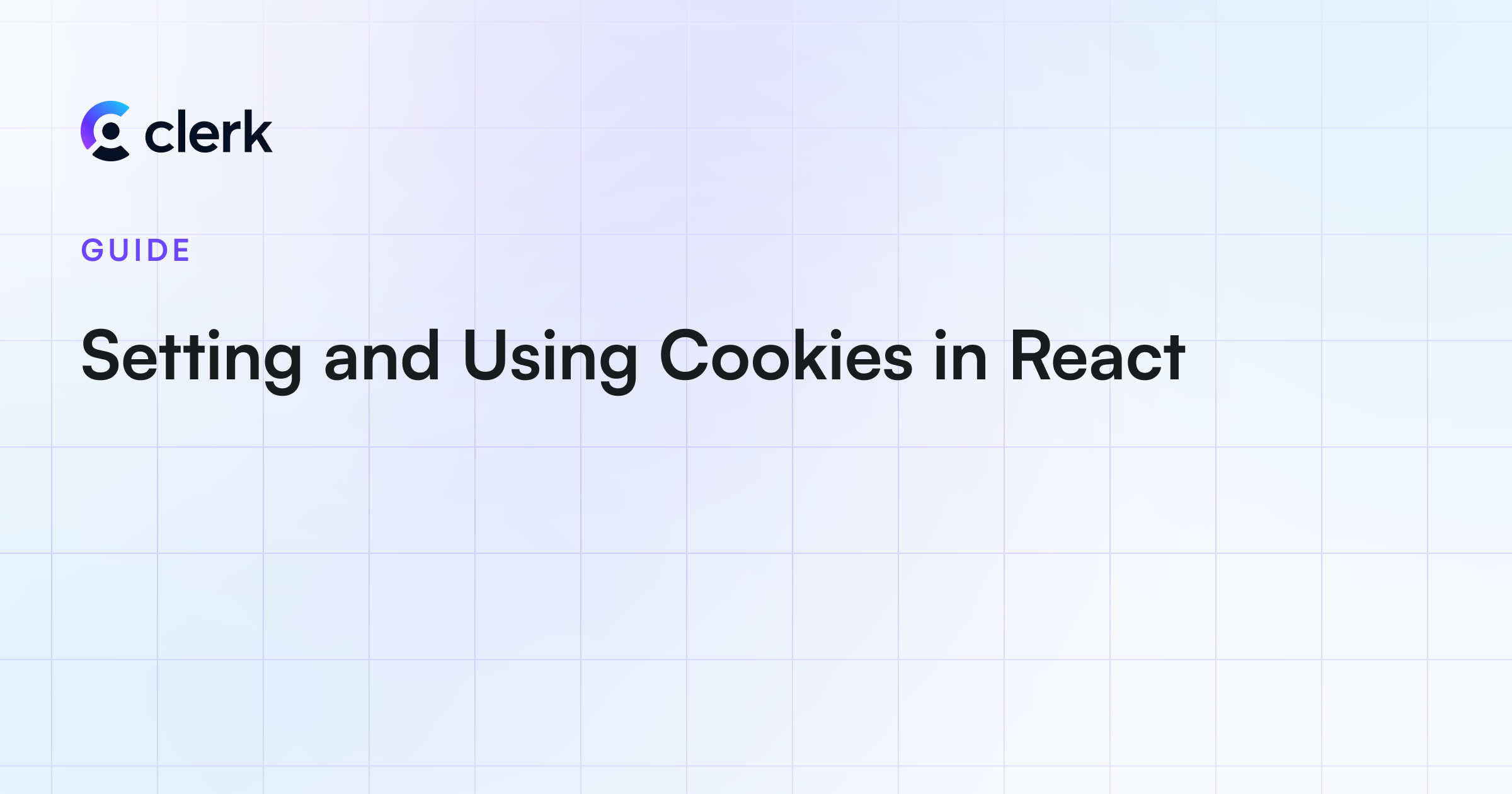
Web cookies consist of data generated by a server and sent to a user's web browser (such as Chrome or Firefox). These small pieces of information are then stored by the website on the user's device. Cookies can be used to keep track of things like items in a shopping cart. If the user leaves the website and comes back later, the items will still be there waiting for them.
They can also be used for security purposes, such as user authentication, and for tracking a user's behavior, which allows websites to personalize the user's experience. For instance, if a user logs out of your site, they can simply log back in without needing to enter their username and password again. It's important to note, however, that cookies can be stolen by hackers who can use them to impersonate the user on your website.
In this article, you'll explore how to set up cookies in a ReactJS application. You'll create a simple login page and use cookies to store information about the user's logged-in session.
What Are Web Cookies?
As mentioned, web cookies are small pieces of data that are stored on a user's computer by a website. They're typically used to store information about the user, such as their preferences, login information, and other data. Cookies are created and stored by the website when the user visits the site. The website can then retrieve the cookies from the user's computer when they visit the site again, allowing the website to "remember" the user and their preferences.
Cookies are typically stored in plain text, which means that they can be read and modified by anyone who has access to the user's computer. Therefore, it's important to use cookies carefully and securely to protect the user's data.
Before you start the tutorial below, make sure you have npm and Node.js installed on your machine. Once this is done, you can begin working on your React application.
Set Up Your React Application
Open a terminal and create a new React application using the following command: npx create-react-app my-app
. Once the application is created, open the directory with your favorite code editor (such as VS Code) and start working on your application. You'll see a bunch of files and folders that look like the image below.
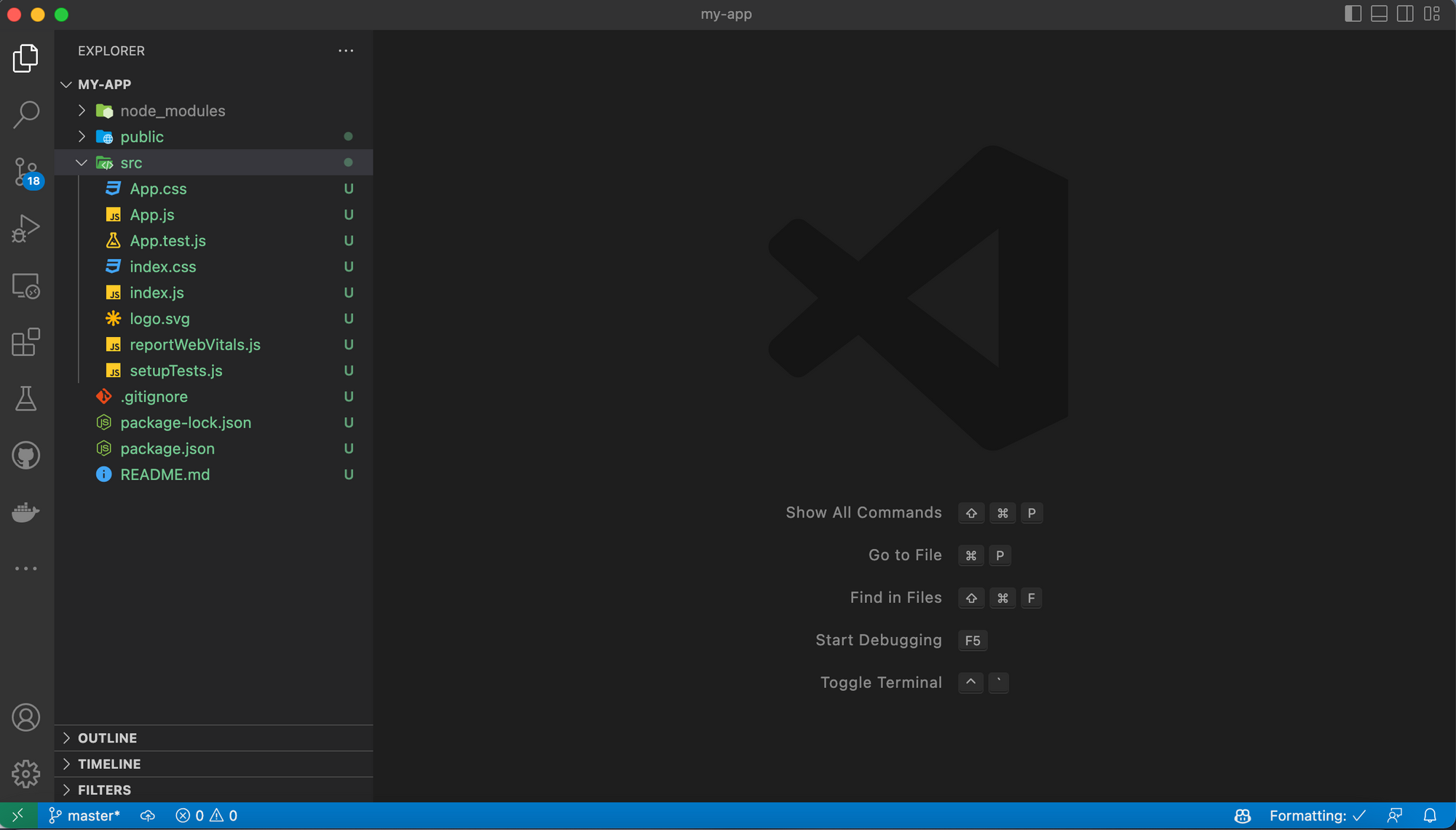
To test that your React application is properly set up, open a terminal and navigate to the my-app
project folder. Start the server by running npm start
; open your web browser and enter the specified port in the terminal (3000
) to view your React application.
By completing these steps, you can verify that your React application is running as expected. You can then proceed with writing code and building your application without any issues. You should see a web page like the image below.
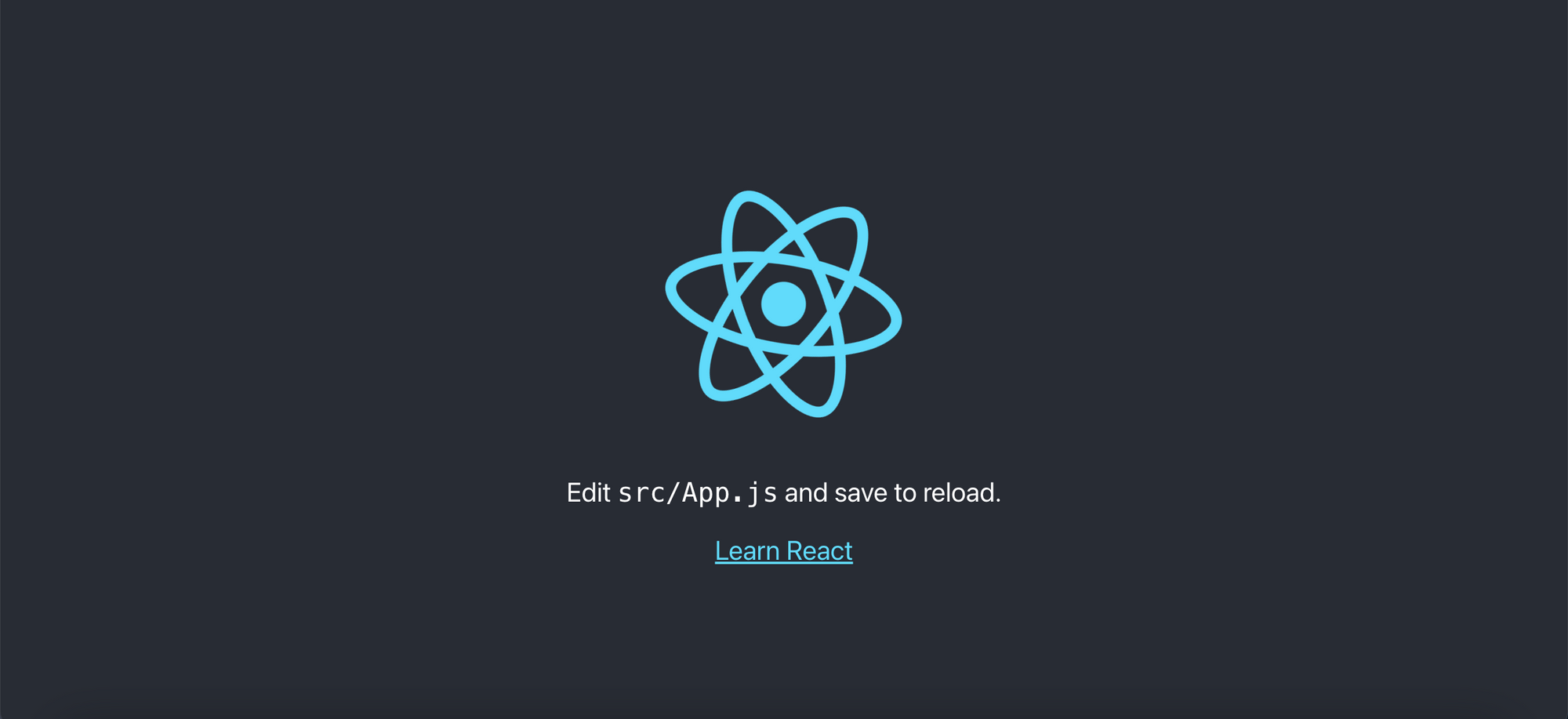
Create a Simple Welcome Page
In your React project, create a new file called WelcomePage.js
inside the src
folder and add the following code inside:
import React from 'react'
export default function WelcomePage() {
return <div>WelcomePage</div>
}
To render the welcome page, first navigate to the App.js
file inside the src
folder. Import the WelcomePage.js
file using the following code:
import WelcomePage from './WelcomePage.js'
Then, inside the return statement, remove everything and add this code:
<WelcomePage />
In the above instructions, you imported the WelcomePage.js
file and used the <WelcomePage />
tag inside the return statement of the App.js
file. This allows the App.js
file to render the welcome page in a web browser. When the page is rendered, you should see a white page with the word "WelcomePage" on it.
Create a Login Page
Create another file inside the src folder called LoginPage.js
and add the following block of code:
import React, { useState } from 'react'
function LoginPage({ onLogin }) {
const [username, setUsername] = useState('')
const [password, setPassword] = useState('')
function handleSubmit(event) {
event.preventDefault()
onLogin({ username, password })
}
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input type="text" value={username} onChange={(e) => setUsername(e.target.value)} />
</label>
<br />
<label>
Password:
<input type="password" value={password} onChange={(e) => setPassword(e.target.value)} />
</label>
<br />
<input type="submit" value="Submit" />
</form>
)
}
export default LoginPage
This LoginPage
component has fields for a username and a password as well as a submit button. When the user fills out the form and clicks Submit, the onLogin
callback function is called with their username and password. The parent component (App
in this case) can then use this information to set the user cookie and render the WelcomePage
component.
Set Up Cookie Handling
To use cookies in your app, you'll need to import the react-cookie library from the React library. This library allows you to set, get, and delete cookies in your app.
First, install the library by running the command npm install react-cookie
in the root folder of your app in the terminal.
Now change your App.js file to match the following code:
import React from 'react'
import WelcomePage from './WelcomePage.js'
import LoginPage from './LoginPage.js'
import { CookiesProvider, useCookies } from 'react-cookie'
function App() {
const [cookies, setCookie] = useCookies(['user'])
function handleLogin(user) {
setCookie('user', user, { path: '/' })
}
return (
<CookiesProvider>
<div>
{cookies.user ? <WelcomePage user={cookies.user} /> : <LoginPage onLogin={handleLogin} />}
</div>
</CookiesProvider>
)
}
export default App
In the code above, the App
component uses the useCookies
hook to manage cookies. The handleLogin
function is called when a user logs in, and it sets a cookie named "user" with the user's information.
The LoginPage
and WelcomePage
components are wrapped in the CookiesProvider
component, which provides a global context for cookies. This allows the WelcomePage
component to access the user cookie and display the user's information.
The App
component uses a ternary operator to decide which component to render based on the presence of the user cookie. If the cookie exists, the WelcomePage
component is rendered. If the cookie does not exist, the LoginPage
component is rendered instead.
Now, if you check your page on your browser, you should see a login form that looks like the image below.
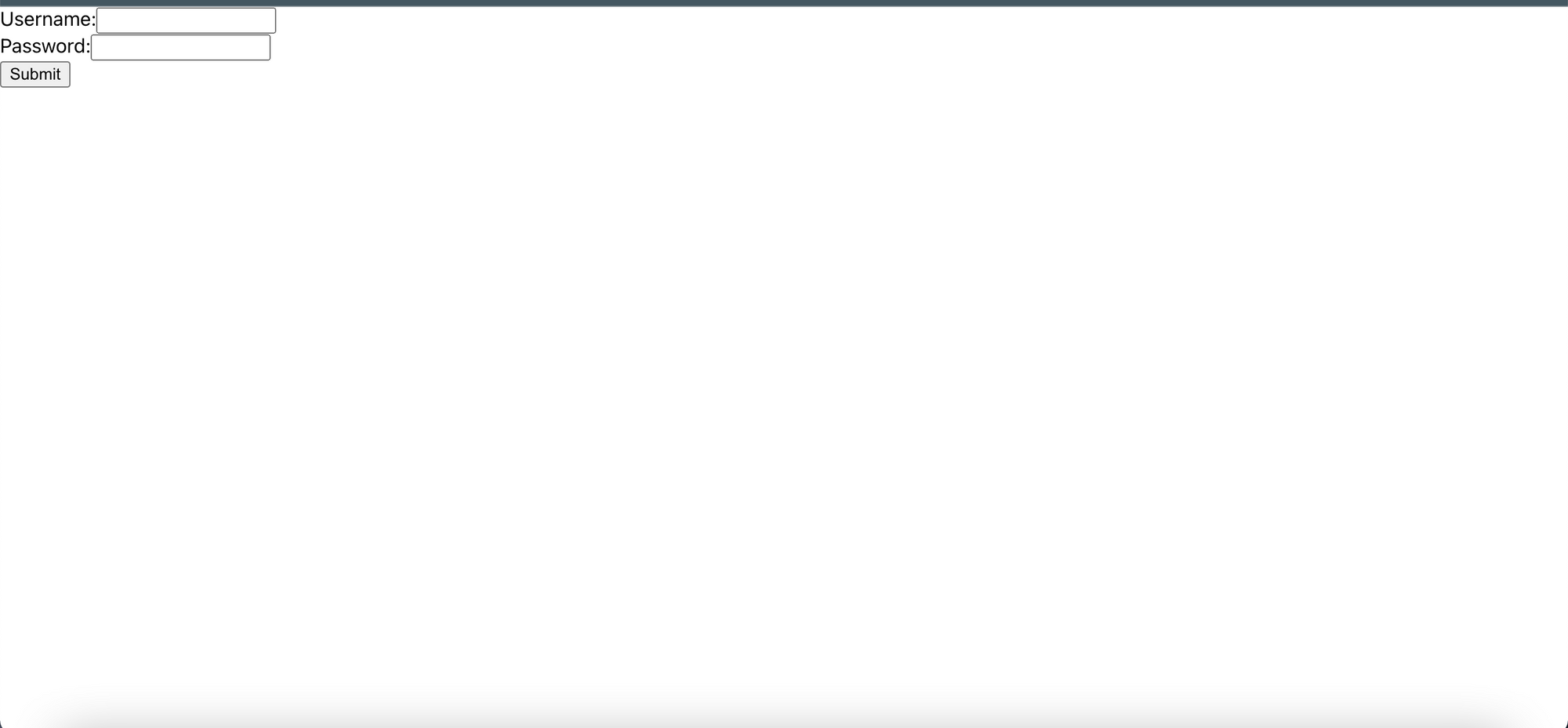
Before you test your application, first change the WelcomePage.js
file to match the following block of code:
import React from 'react'
function WelcomePage({ user }) {
return <h1>Welcome, {user.username}!</h1>
}
export default WelcomePage
This WelcomePage
component simply displays a welcome message with the user's username. It receives the user object as a prop, which contains the user's username and password. The parent component (App
in this case) passes the user object to the WelcomePage
component when WelcomePage
is rendered.
Test Your Application
To test your application, go to the browser and fill in the login form with your username and password. When you click Submit, the welcome page should be displayed with the words "Welcome" followed by your username. This indicates that the login was successful and the WelcomePage
component was rendered. It should look like the image below.
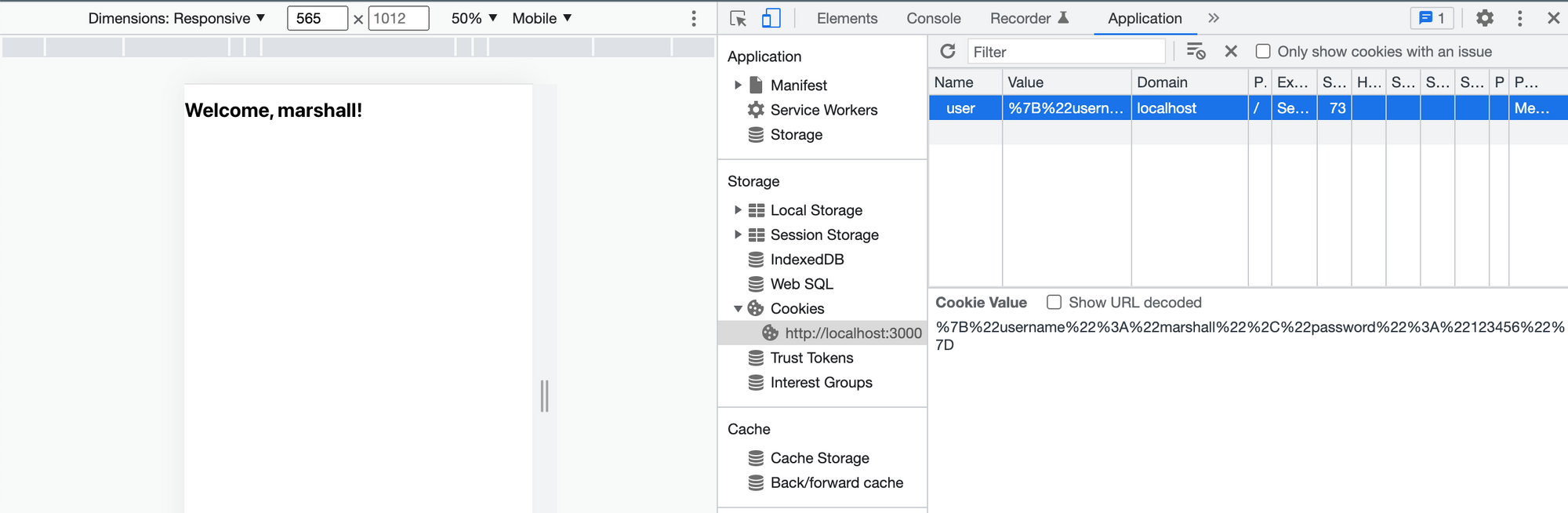
To check if a cookie has been set in your Chrome browser, you can use the developer tools. Open your browser, and if you're on Chrome, go to the page where the cookie is set by pressing F12 on your keyboard to open the developer tools.
Click on the Application tab in the developer tools. In the left panel, expand the Cookies section and click on the domain where the cookie is set—in this case, it's http://localhost:3000
. In the right panel, you should see a cookie that has been set for the domain like in the image above.
You can find the complete code for this tutorial on GitHub.
Conclusion
In this tutorial, you learned how to create a login page and a welcome page in a React app and how to store the user's login information in a cookie. You also learned how to only display the welcome page if the user is logged in and how to check if a cookie has been set in the Chrome browser.
Using cookies in a React app can make the login process more user-friendly as it allows users to access the welcome page without having to enter their login information every time they visit the page.
Clerk provides an easy way to add authentication and user management to your application. Clerk handles session management, including setting cookies on your behalf, saving you time and effort. This allows you to focus on building the features of your app without having to worry about implementing the authentication and session management features from scratch. Give Clerk a try by signing up today.
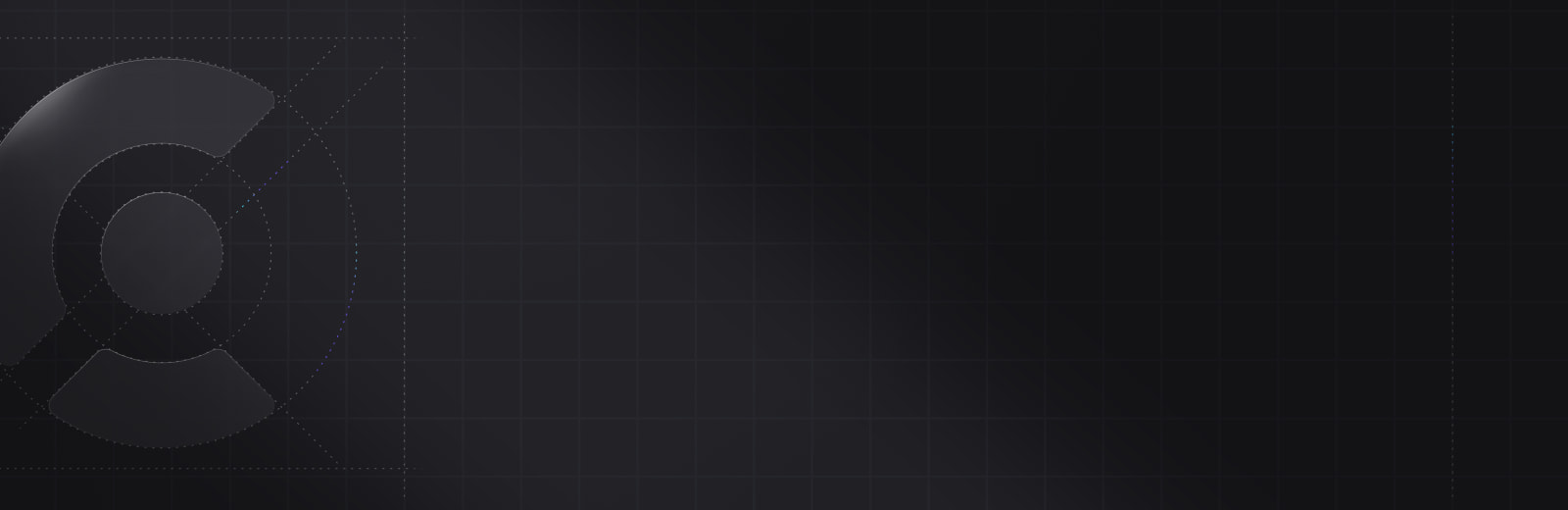
Ready to get started?
Sign up today