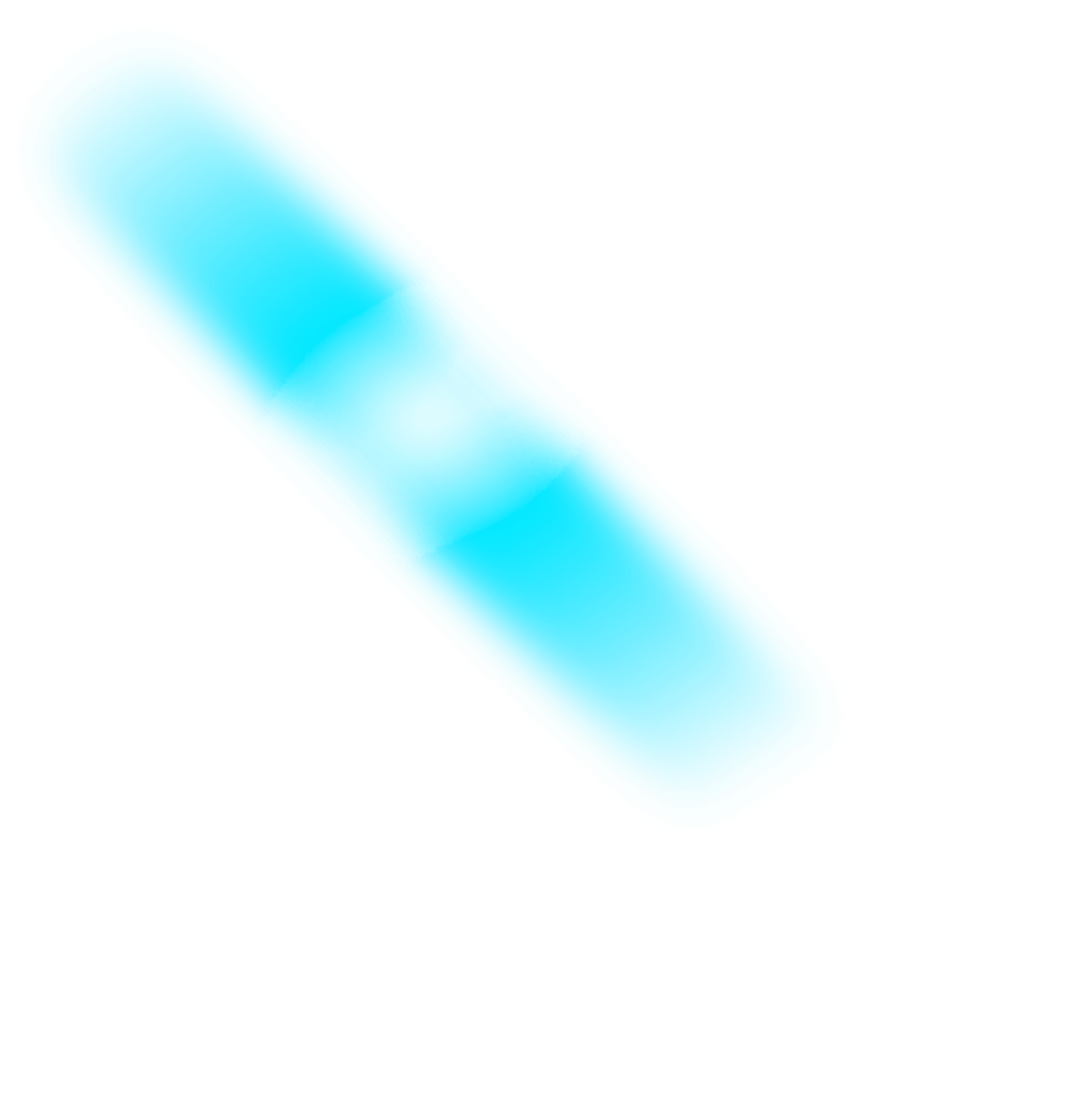
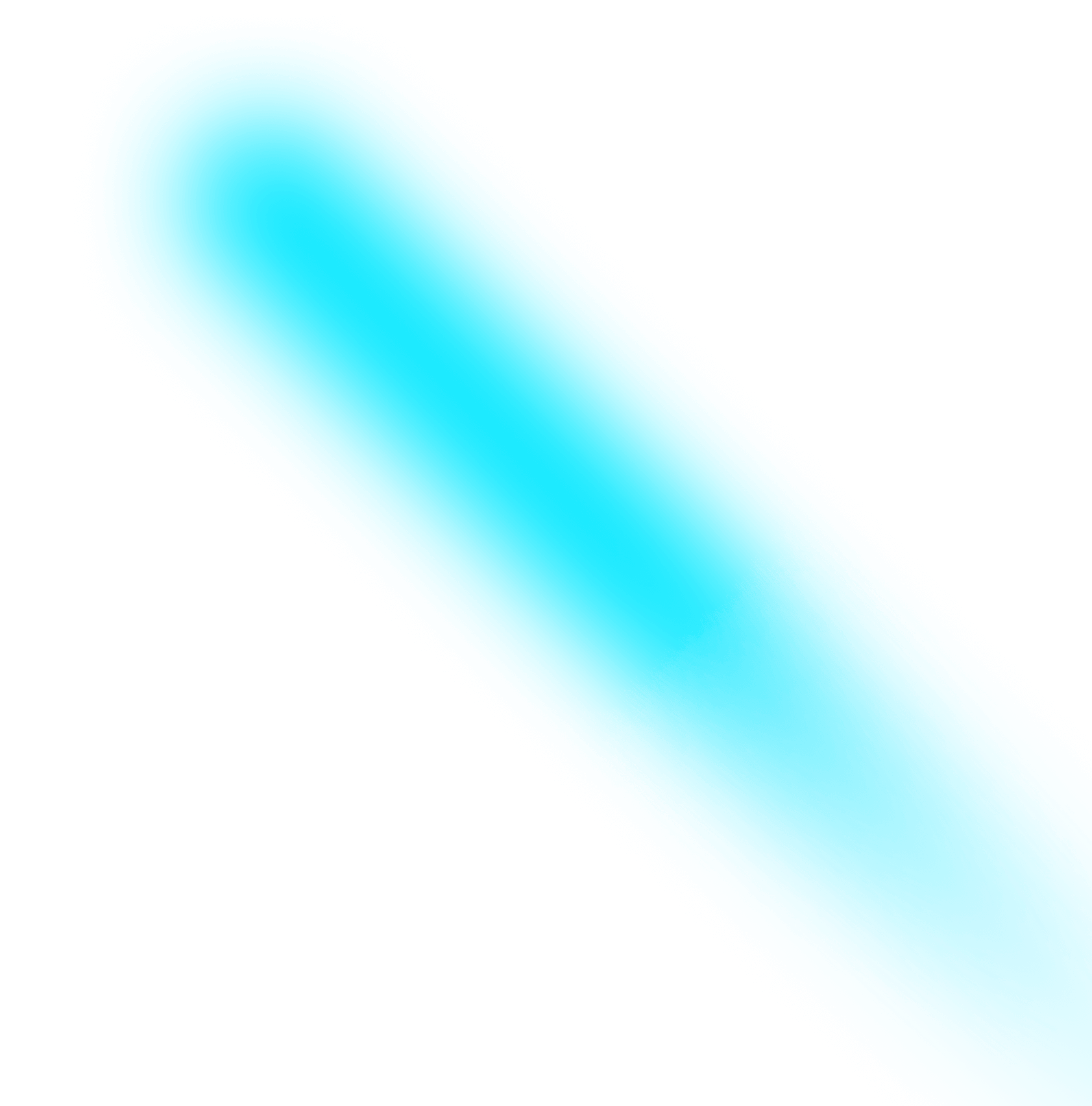
React Authentication
Authentication optimized for React
Implement authentication and user management the React way - with hooks and components.
Trusted by fast-growing companies around the world
UI Building Blocks
Beautiful prebuilt components to authenticate and manage your users
UI components for secure user sign-in, sign-up, profile management, organization management, and more.
Clerk components work quickly out-of-the-box and can be customized to perfectly match your brand.
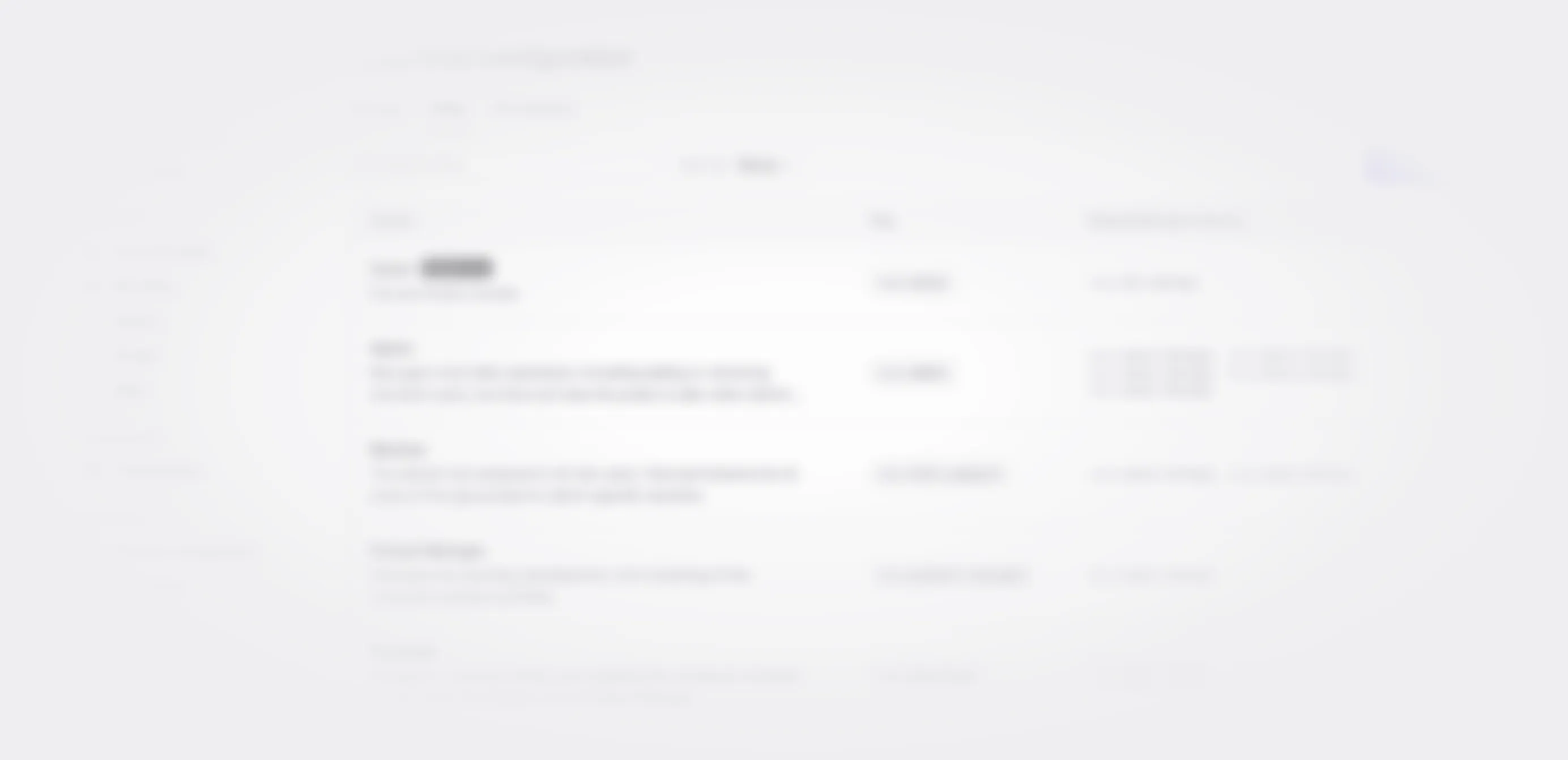
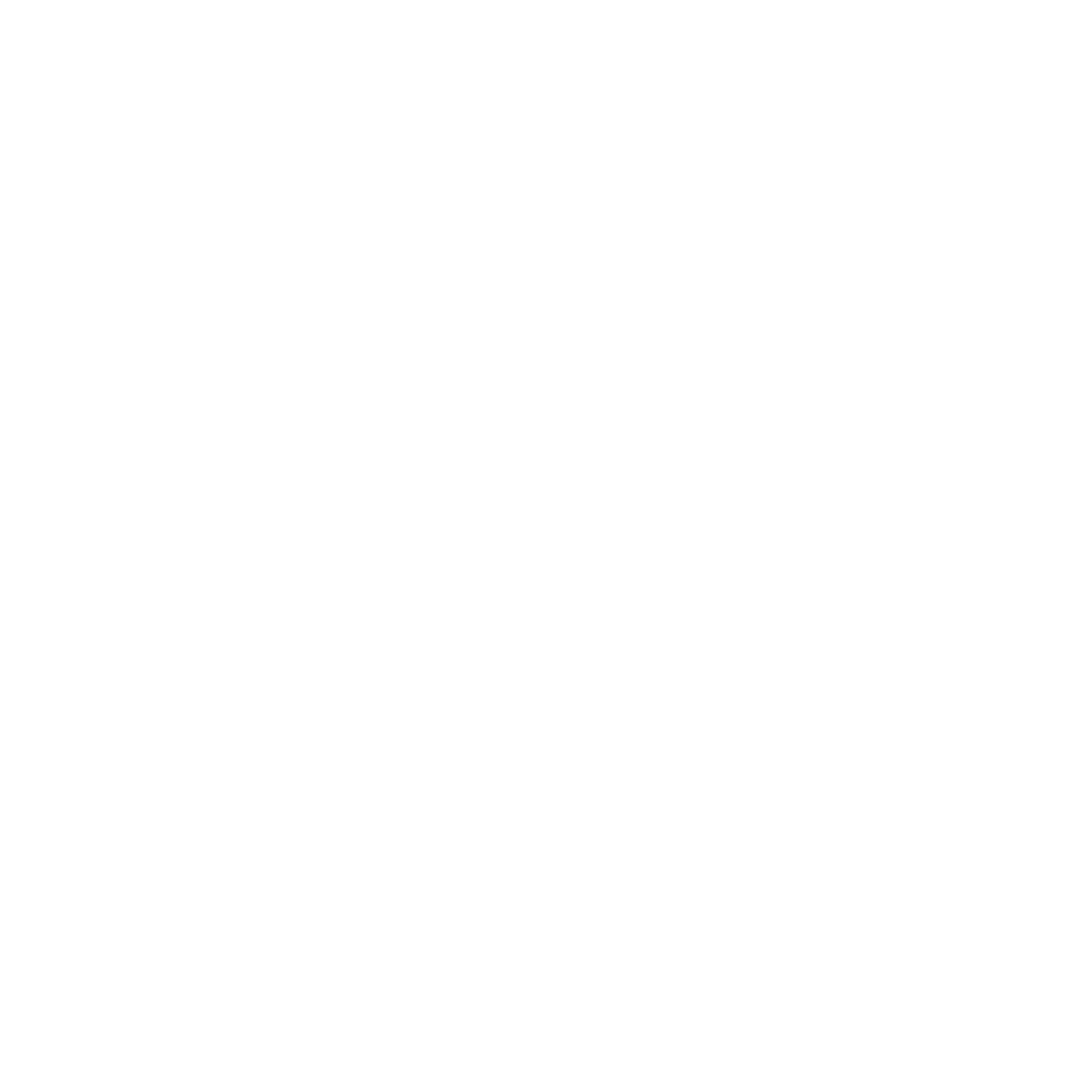
Hooks and components
Everything you need for authentication
Clerk equips you with the tools and features necessary to implement the authentication experience your users deserve.
Access user data anywhere
Clerk efficiently handles user state management, giving you access to fresh user data wherever you need it.
useUser()
Protect your pages
Use control components like <SignedIn />
, <SignedOut />
, and <RedirectToSignIn />
to secure your pages and redirect unauthenticated users.
import {
SignedIn,
SignedOut,
RedirectToSignIn
} from '@clerk/clerk-react'
const ProtectPage = () => {
return (
<>
<SignedIn>
{children}
</SignedIn>
<SignedOut>
<RedirectToSignIn />
</SignedOut>
</>
)
}
export default ProtectPage
Role-based access control
Define and assign permissions from the Clerk dashboard then conditionally render components based on the user’s access rights.
import { Protect } from '@clerk/clerk-react'
export default function ProtectPage() {
return (
<Protect
permission="org:invoices:create"
fallback={<p>You don't have permission</p>}
>
{children}
</Protect>
)
}
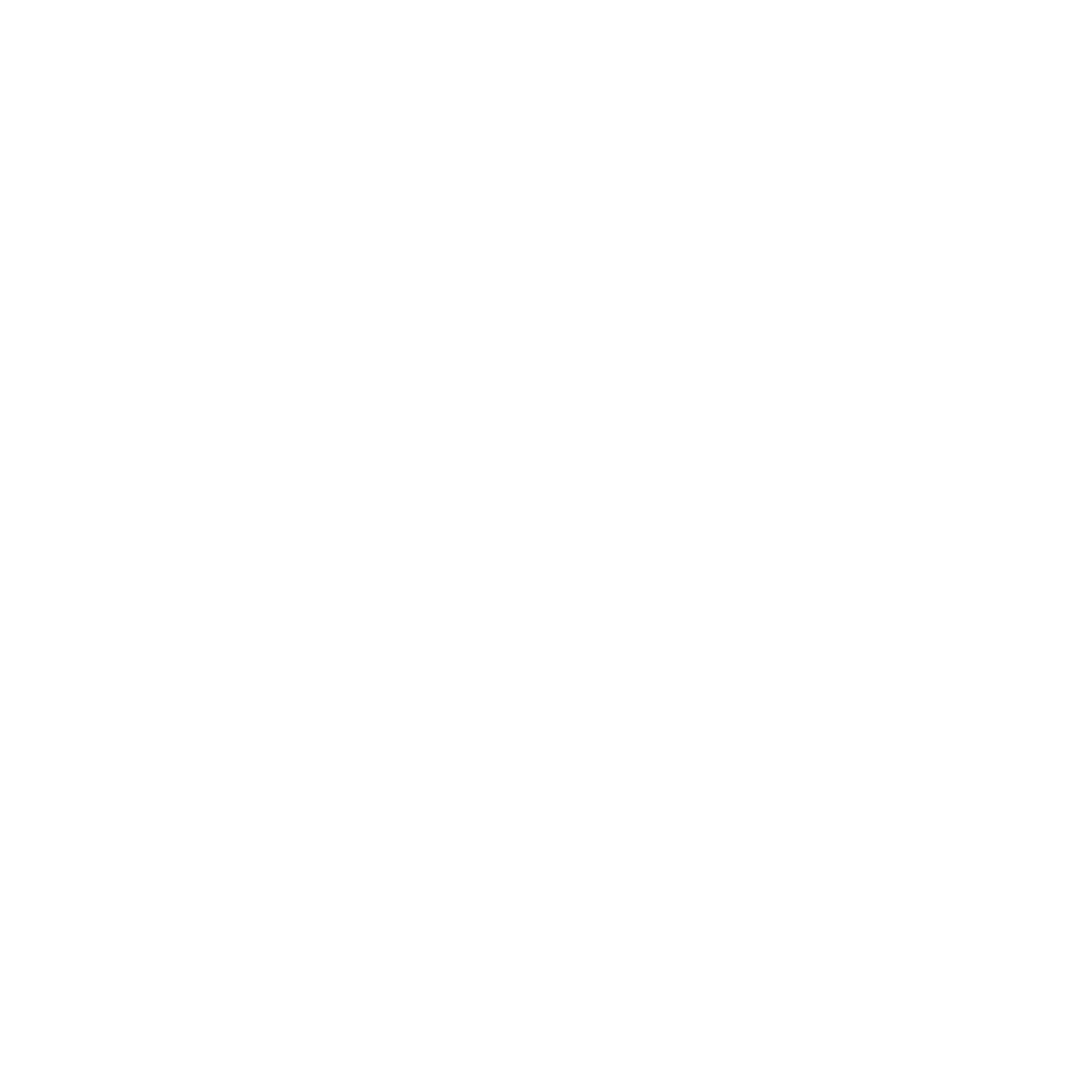
Complete session management
Don't touch a JWT, unless you want to. Clerk manages the entire session lifecycle, providing essential features like session revocation and active device monitoring.
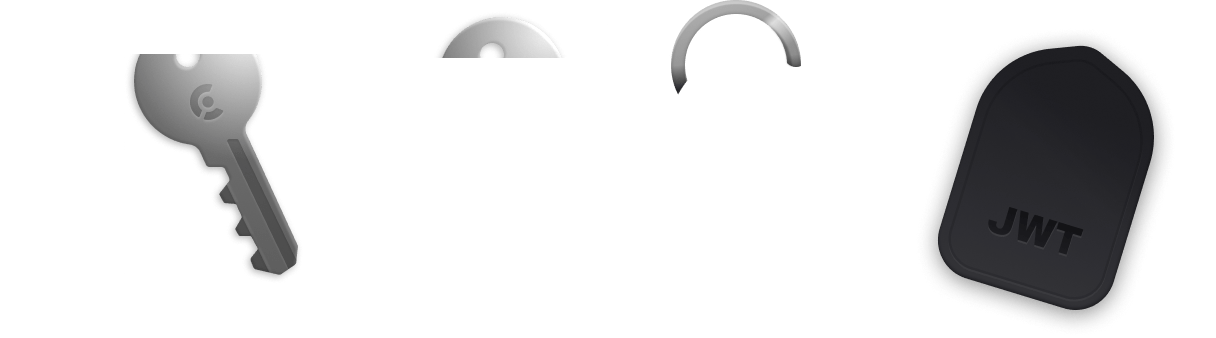
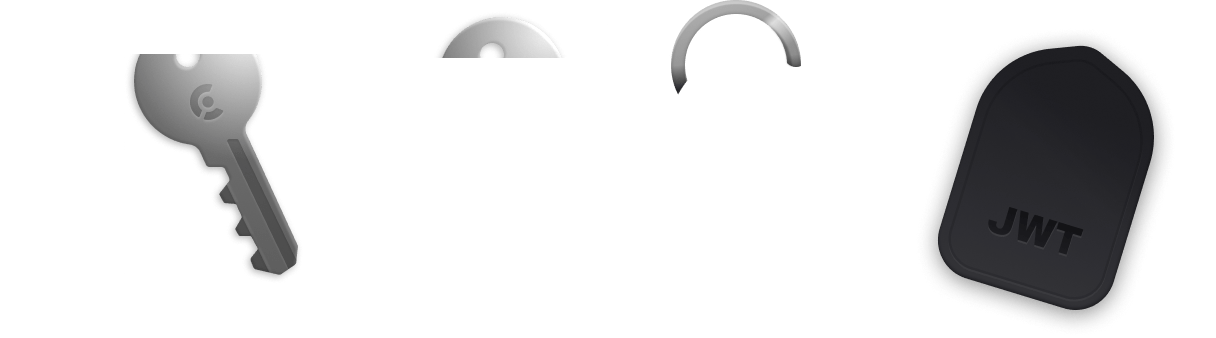
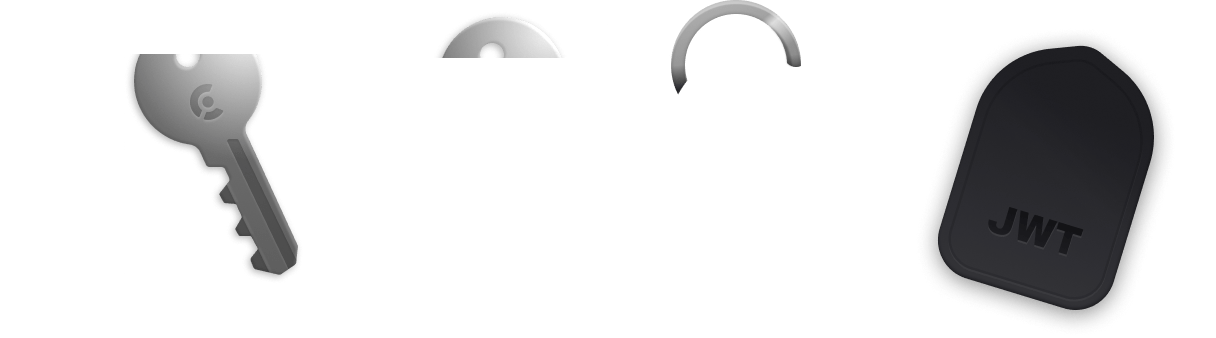
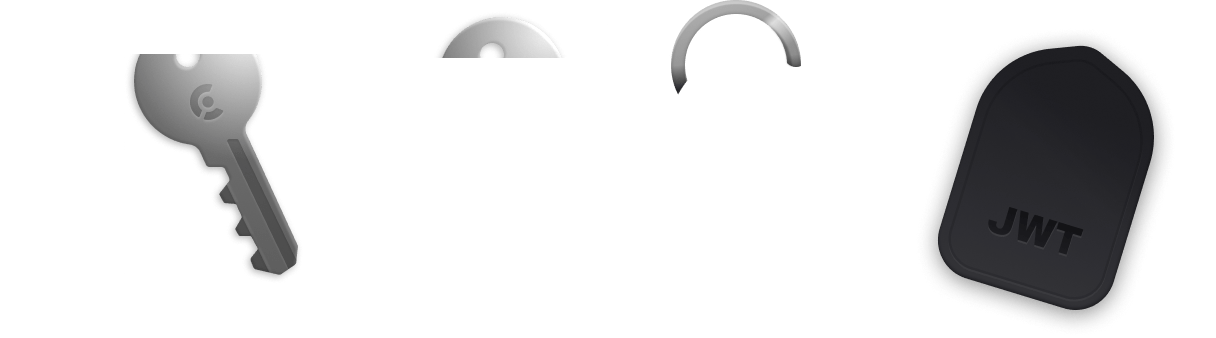
Hardened user security from day one
Never compromise on user security or privacy to launch quickly. Protect your users from day one with MFA and new device alerts. Stop fraudulent bots in their tracks with Clerk's anti-abuse heuristics.
React SDKs
Build with your choice of framework
Clerk's React SDKs are purpose-built to integrate naturally with each framework's patterns and features. Choose one to get started.
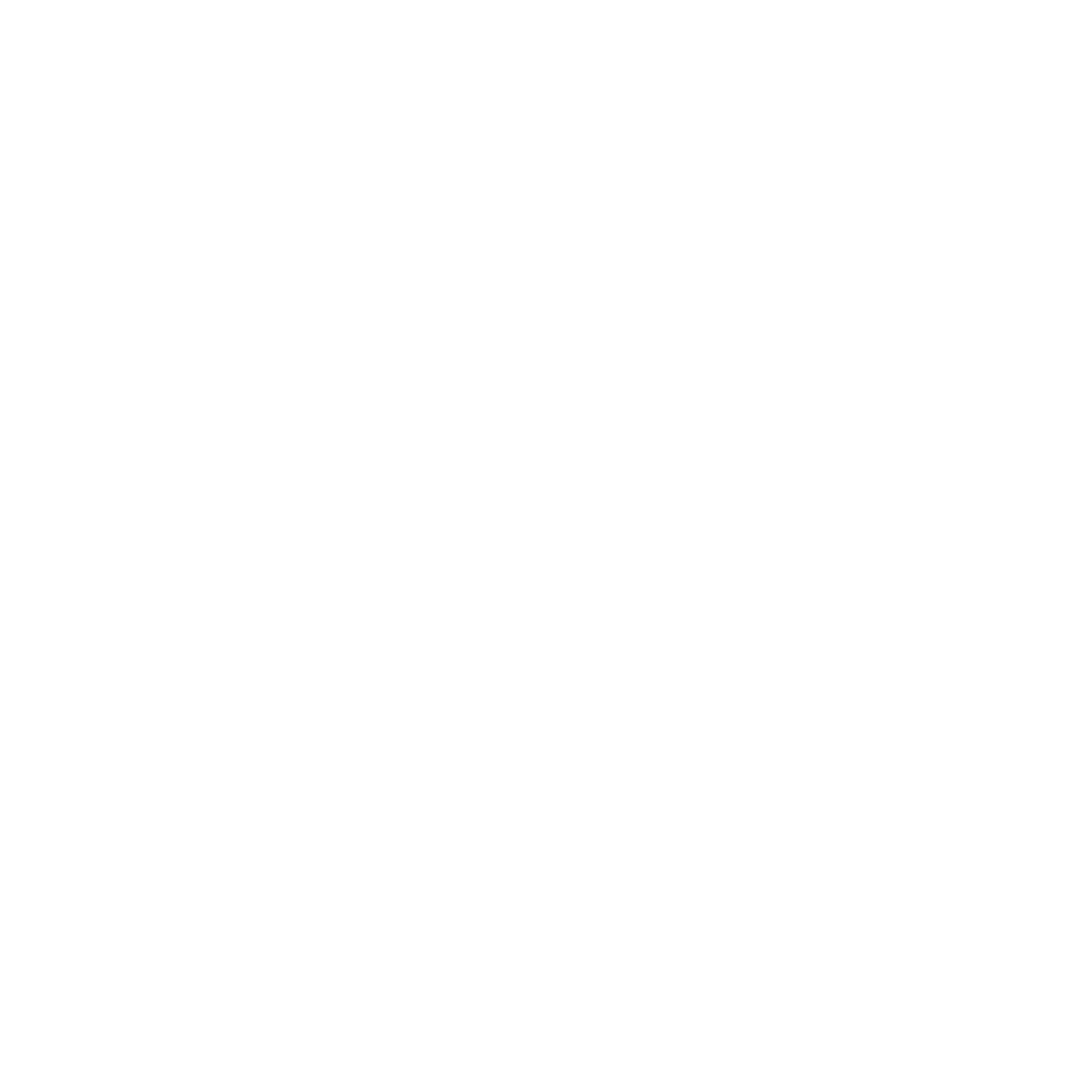
Backend SDKs
Authenticate on any backend
Clerk's backend SDKs offer authentication helpers to easily access important data like the currently active user's ID.
React framework backends
Standalone backends
Backed by experts
Endorsed by engineering leaders and technical investors
The best practices built-in to their <SignIn/> and <UserProfile/> components would take months to implement in-house, yet no sacrifice is made in terms of Enterprise extensibility or customization to your brand.

- Name
- Guillermo Rauch
- Role
- CEO
- Company
- Vercel
Clerk feels like the first time I booted my computer with an SSD. It’s so much faster and simpler that it changed how I do things.

- Name
- Theo Browne
- Role
- CEO
- Company
- Ping Labs
After spending many hours on auth issues that seemed simple (but were not), we moved to Clerk and all that burden was lifted. We kind of wish we’d made that decision earlier.

- Name
- Julian Benegas
- Role
- CEO
- Company
- BaseHub
We’re big admirers of what the @ClerkDev team are building and looking forward to working more closely with them.

- Name
- Patrick Collison
- Role
- CEO
- Company
- Stripe
We were able to ship MFA, SSO, and SAML for our customers in a fraction of the time. Now, we have improved security and must-haves for enterprise prospects.

- Name
- Dan Farrelly
- Role
- CTO
- Company
- Inngest
Clerk’s integration gives Supabase developers another incredible option for handling authentication. And the Clerk team are a pleasure to work with.

- Name
- Paul Copplestone
- Role
- CEO
- Company
- Supabase
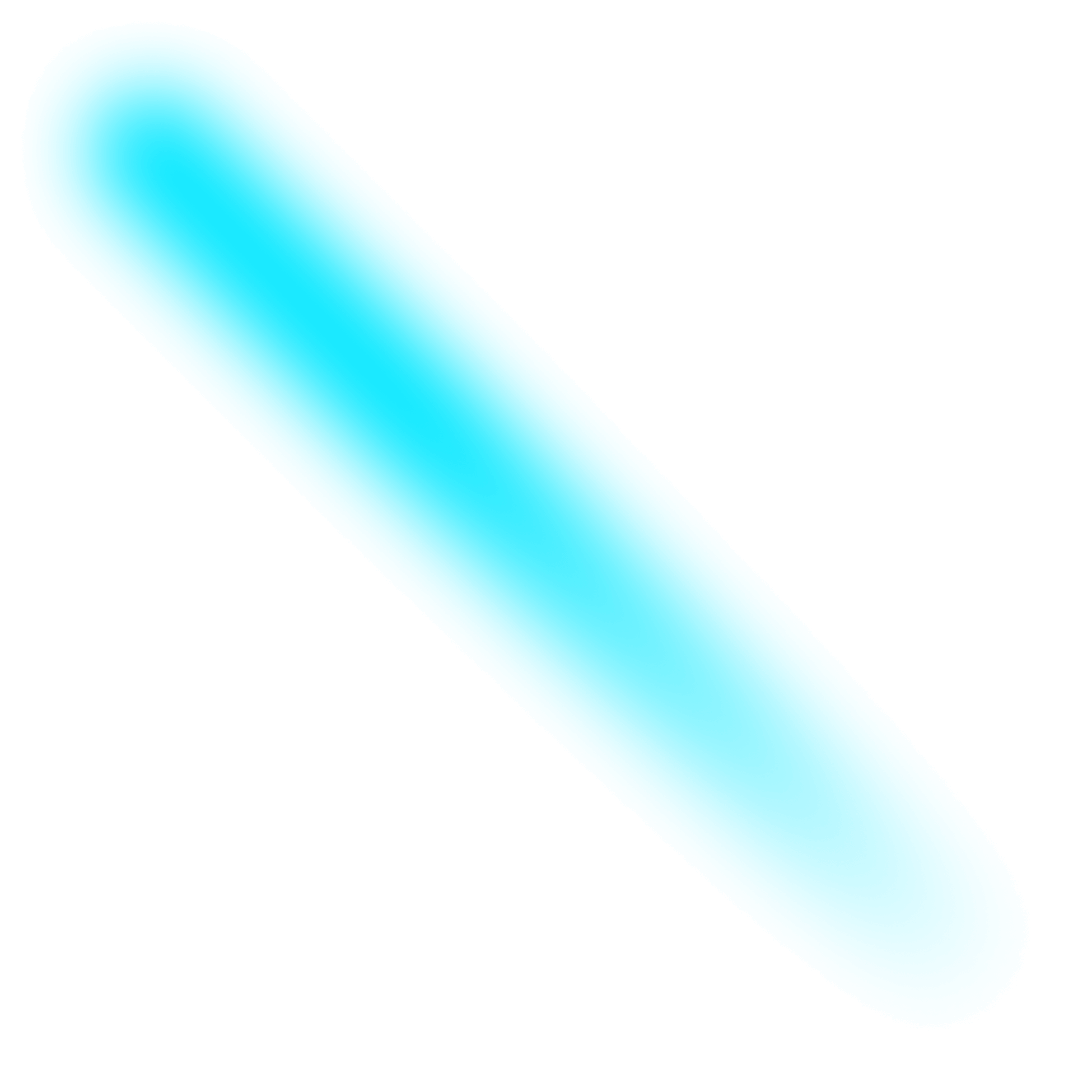
Sophisticated security
Take the security burden off your shoulders
Security is Clerk's most important responsibility and the central focus of every feature we build. We undergo rigorous security testing and maintain certification with leading industry standards.
Secure, private, and compliant. Always.
SOC2 Type II
HIPAA
CCPA
Comprehensive user management
Much more than authentication
Authentication is only the beginning. Clerk gives you everything you need to manage your growing user base - from authorization and organization management to a powerful admin dashboard.
Build team-based features quick with Organizations
Enable your users to create and manage teams, invite team members manually, or set up auto-invites for teammates who share a company email domain.
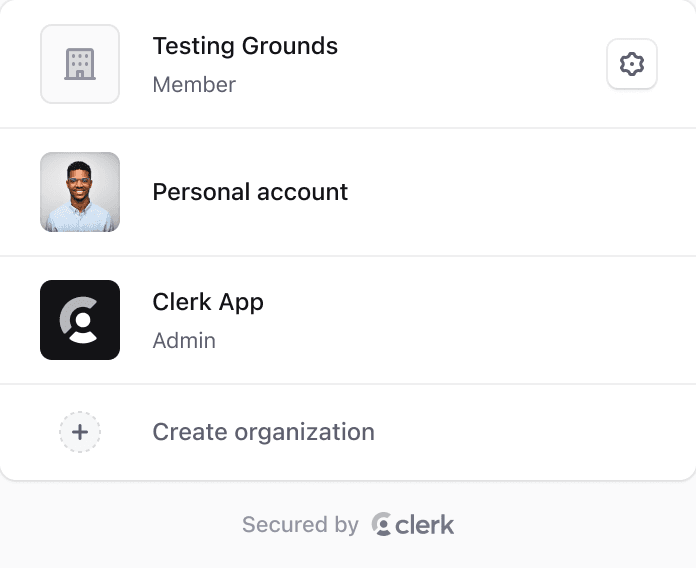
Manage and support users from the Clerk dashboard
Monitor, manage, and support users directly from the Clerk dashboard. It's the most powerful user management back office you never built.
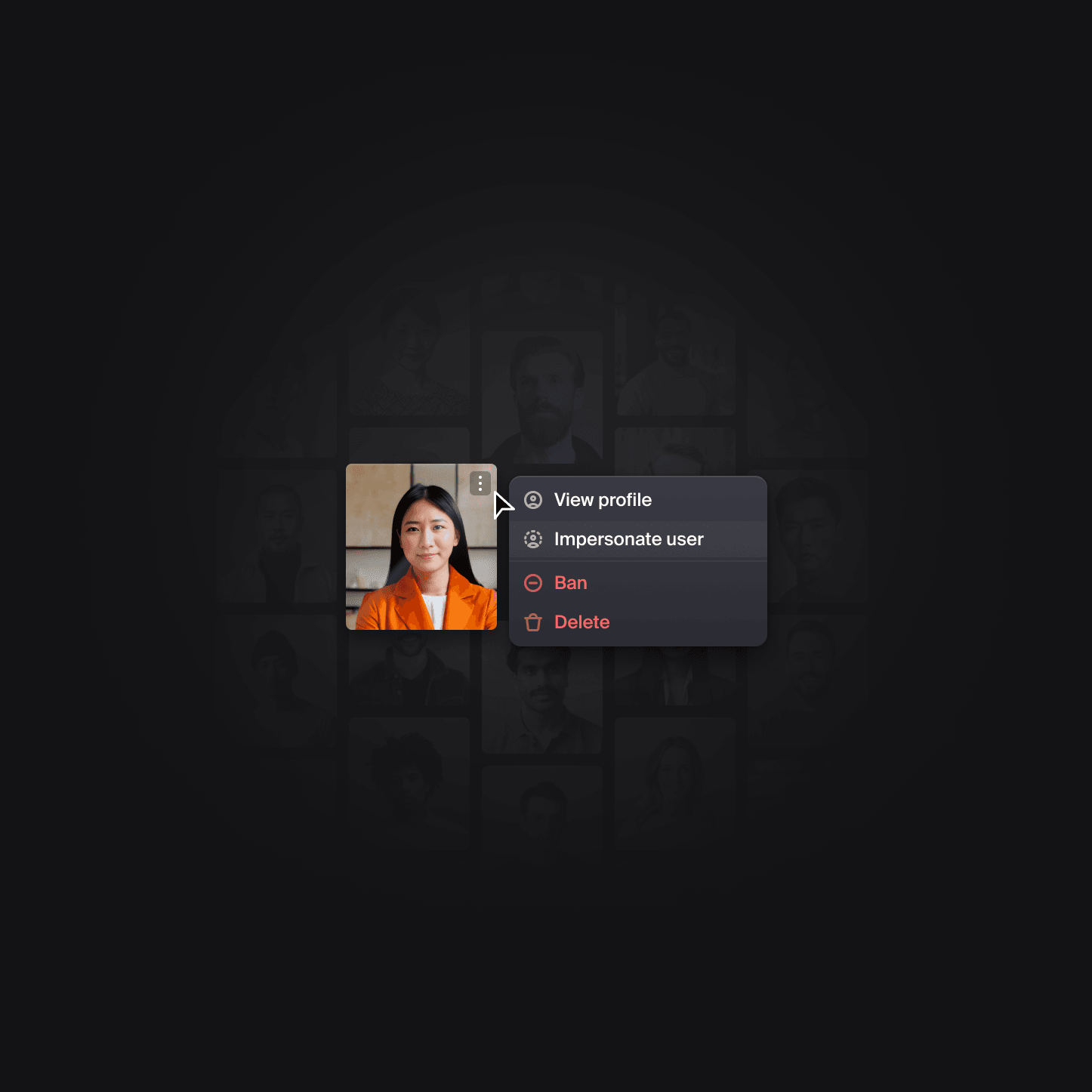
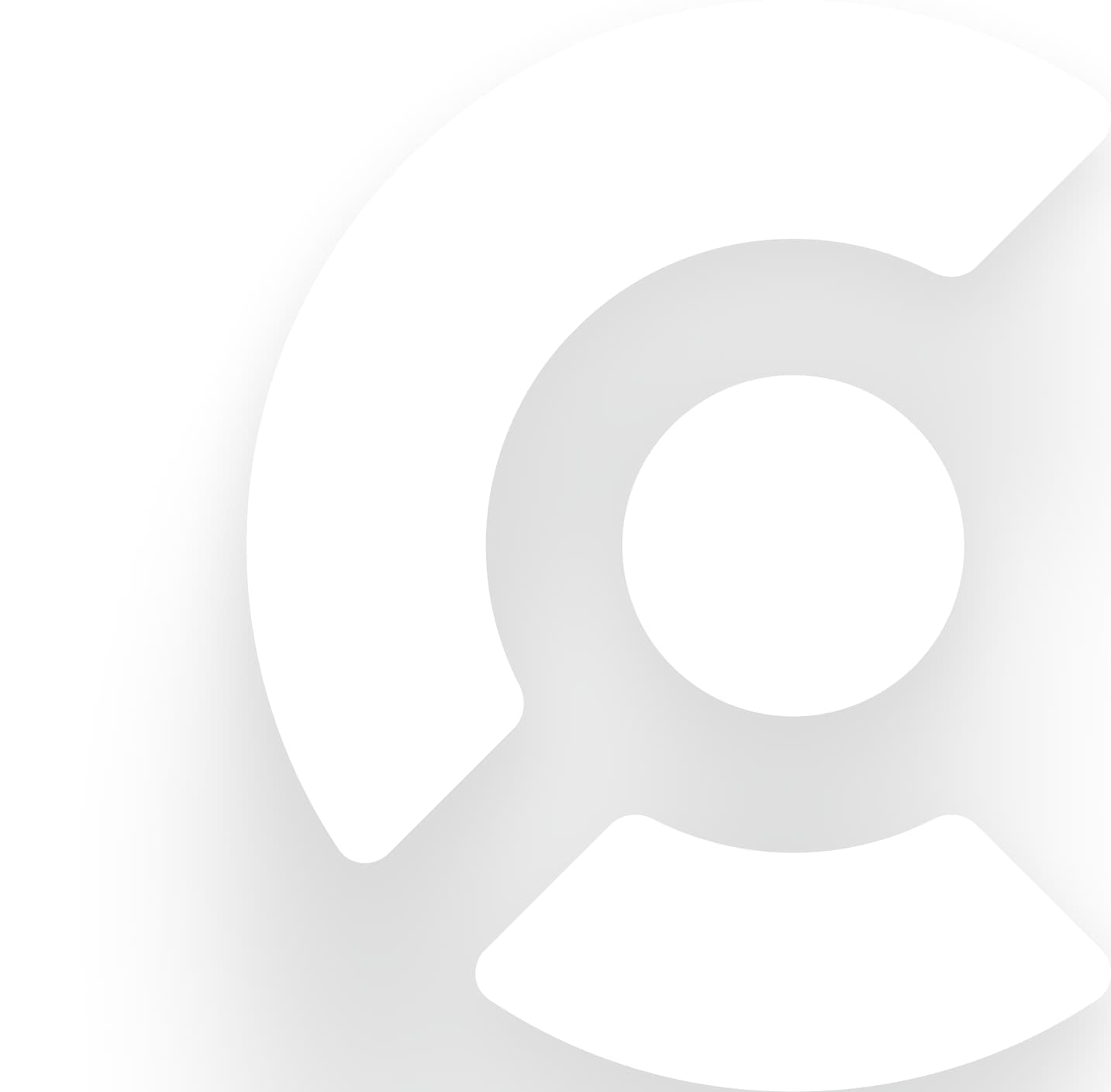
Add React authentication with a few lines of code
Try Clerk for yourself. Free for your first 10,000 monthly active users and 100 monthly active organizations. No credit card required.
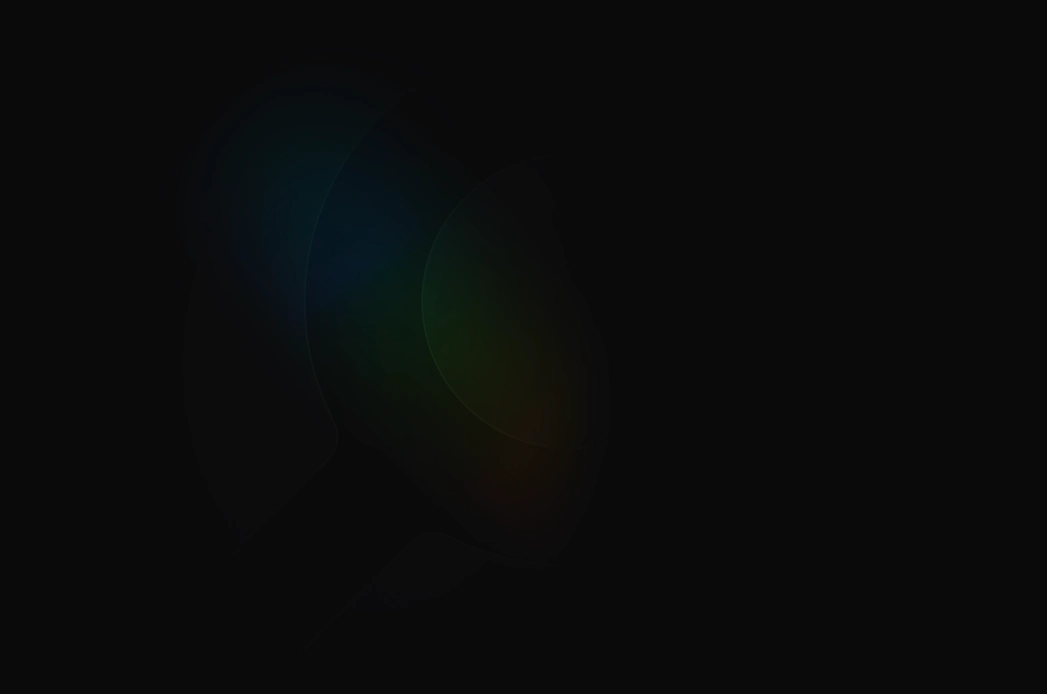
Frequently asked questions
How does Clerk work with React?
Clerk’s React SDK provides prebuilt components, hooks, and helpers that make it easy to add authentication, user management, and session handling to your React app. It’s built on top of our core JavaScript SDK, so you get powerful primitives with a developer-friendly React interface. You can use our components out of the box or fully customize them to match your UI. Refer to the quickstart guide to get started.
How easy is it to install and configure Clerk’s React SDK?
Run npm install @clerk/clerk-react
, set up your Clerk publishable key as an environment variable, wrap your app with <ClerkProvider>
, and you’re ready to go. The whole setup takes a few minutes.
How many prebuilt React components does Clerk provide?
Clerk provides a comprehensive set of prebuilt React UI components for authentication and user management, including components for sign-in, sign-up, user profile, B2B organization management, SaaS billing and more. See the full list of UI and control components we provide.
Can I customize Clerk React components?
Yes, you can customize Clerk React components to suit your application’s design and functionality. Clerk offers pre-built components like <SignUp />
and <SignIn />
that are easily customizable. You can modify their appearance with custom CSS, override default styles, and pass props to adjust behaviour, ensuring they perfectly match your brand. See our customization guide.
Do I have to use Clerk React components?
We recommend using Clerk’s components for most authentication needs, as they provide a flexible and efficient way to manage user and session states within your React application. However, if you require truly custom control over your authentication flows, you can use Clerk hooks to create custom sign-in and sign-up processes, retrieve user data, and check authentication status, offering flexibility beyond pre-built components.
How many single sign-on (SSO) options can my React app support with Clerk?
Clerk supports all major SSO protocols (SAML, OAuth, OpenID Connect), letting you integrate with providers like Google Workspace, Microsoft Entra ID (Azure AD), Okta, and more, directly from your React app. Social logins like Google, GitHub, LinkedIn, and Slack are also available out of the box. The Free plan supports up to 3 enterprise SSO connections, while the Pro and custom plans offer unlimited SSO connections.
Can I use Clerk with other React libraries like React Router?
Yes, you can use Clerk with other React libraries like React Router. Our @clerk/clerk-react
SDK supports React applications and you can integrate React Router using their library mode to handle routing. If you’re using React Router in a project you can use the @clerk/react-router
SDK, which is designed to integrate directly into the framework’s conventions without requiring @clerk/clerk-react
.
Why do default JWTs expire every 60 seconds?
Default JWTs expire every 60 seconds to reduce the risk of outdated or compromised tokens being used. Clerk automatically refreshes the JWT behind the scenes using the session cookie, ensuring your app remains secure without requiring manual intervention or any action from the user.
How do I migrate to Clerk from another auth provider?
Clerk supports two main migration strategies: basic import/export and trickle migration. With import/export, you can batch import users using Clerk’s CreateUser
API and export tools. This is ideal for one-time migrations, but you’ll need to manage timing and API rate limits carefully. Trickle migration lets you run your existing auth system alongside Clerk and gradually transition users as they sign in, reducing risk and downtime. We provide an open-source CLI tool to assist with imports and supports secure password hash upgrades when needed.
Can I export my users’ data from Clerk?
Yes, you can export user data programmatically using our GetUserList
API or the getUserList()
method in the JavaScript SDK. Additionally, admins and workspace owners can export users directly from the Clerk Dashboard as a CSV file, including hashed passwords. The dashboard also provides detailed export history logs for full visibility and traceability of all exports.
Why would I trust Clerk with my users’ data?
We’re SOC 2 Type II compliant (audit report available to customers) and we provide a clear Data Processing Agreement and maintain a detailed list of subprocessors to ensure transparency. Our infrastructure is hosted on Google Cloud Platform and we’re safeguarded by Cloudflare against attacks. We also enforce rigorous internal access controls and strict data management policies to keep your data secure.
Is Clerk secure and privacy compliant?
At Clerk, security and privacy are core principles. We encrypt all data both in transit and at rest, enforce rigorous access controls, and comply with key privacy regulations such as GDPR, CCPA, and the EU-U.S. Data Privacy Framework. See our Data Privacy Framework and Data Processing Agreement.
How can I handle localization or internationalization with Clerk in React?
Clerk provides localization through the @clerk/localizations
package, offering pre-defined translations in over 30 languages, including Arabic, German, Spanish, French, and Japanese. You can also create custom translations to modify text in Clerk components. Note that this feature is currently experimental.
Can I use Clerk with React Native?
Yes, you can use Clerk with React Native through our dedicated Expo SDK.