The Ultimate Guide To JSON Web Tokens (JWTs) and Token-Based Authentication
- Category
- Insights
- Published
JSON Web Tokens, more commonly known as JWTs, are encoded and cryptographically signed data that allows for the secure transfer of information.
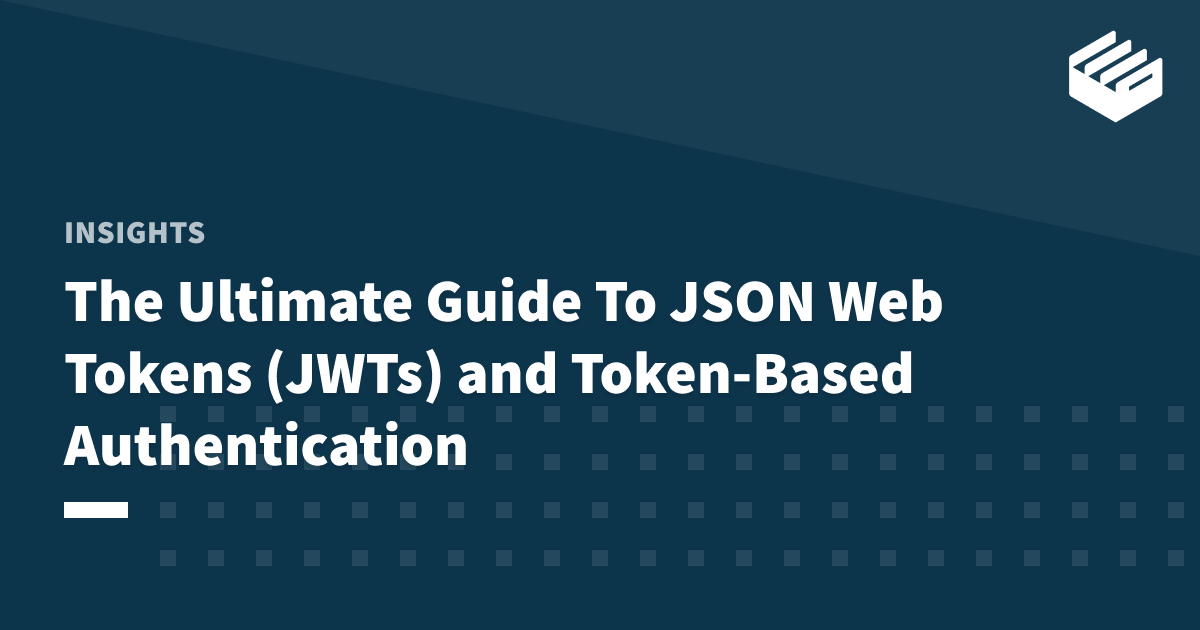
Security has become a primary concern in today's digital world. With almost every business process going digital, individuals and businesses must ensure fool-proof security and keep their data safe. Not just their information — they also need to protect their clients' data from any digital fraud. That's where JavaScript object notation (JSON) web tokens (JWTs) and JWT token authentication come in handy.
JWT is a compact and URL-safe way of transferring claims or information between two parties. The JWT authentication method makes sure that the information reaches the party it was intended for.
If you want to know all the ins and outs of this token-based authentication method, keep reading to find out how it works, what a JWT token is, its benefits, and much more.
Understanding User Authentication
User authentication allows a device to authenticate the identity of someone trying to connect to a network. Typically, it requires the user to sign-up with a unique ID and password, which they can use to sign in later. This way, the computer recognizes the user and lets them in after the authentication process instead of asking "who are you?" every time they sign in.
Simply speaking, the user authentication process allows a user to access their account while blocking all the unauthenticated entries from the same account. The user's ID and password serve as their identity.
When your system's user authentication process isn't secure, it increases the chances of cybercriminals breaching into your system and reaching essential data.
Types of Authentication
To access any network, the user needs to provide the information secured between the network's server and the user, such as a pin, code, or password. This information is referred to as an authentication factor. There are several ways a server ensures user authentication, including the following.
Multi-Factor Authentication
A multi-factor authentication method verifies users' identities via multiple authentication methods. For example, when users enter their ID and password, they won't access the resources directly. Instead, they must verify themselves via a one-time link or a security code sent to them through text messages or emails.
The multi-factor authentication strategy ensures multiple layers of protection over the user's account and private data. Even if a hacker determines any users' ID and password, they also have to go through additional authentication methods — or else, they will be denied access.
API Authentication and API Tokens
Application programming interface (API) authentication is the process of verifying the identity of users trying to get access to the resources on a server. The authentication process includes the use of API tokens.
API tokens are sort of a unique identity of a user or an application trying to access a service. When an application wants to access a service, it generates an API token for the application that it can use when requesting that particular service. Later, when the application makes an access request, the service matches the token provided by the app to the one it had stored to authenticate.
Simply put, an API token works just like a username and password combination. However, the API tokens provide a second layer of security, and the users have substantial control over each action and transaction.
Token-Based Authentication
Token-based authentication is a process that allows a user to verify their identity through a unique access token. As long as the token remains valid, the user can access the website or app from which they received the token. They don’t need to re-enter their credentials, meaning their username and password, each time they try to access the same website or app.
The signed tokens are just like stamped tickets.
What Are JSON Web Tokens (JWTs)?
The compact method of JWTs is an open standard for the safe transmission of information — encoded as a JSON object — between two or more parties.
For example, if you want to sign in to Tinder with your Facebook profile, Tinder will contact Facebook's Authentication server and ask for your username and password. As soon as Facebook's server verifies your credentials — your username and password — a JWT will be created and sent to you. Tinder gets this JWT and allows you to access its services.
What To Know About Token-Based Authentication
Token-based authentication is a process that ensures the security of applications by using signed tokens as a form of verification.
Tokens, like JWTs, eliminate the need for contacting any third-party service for the authentication process. Instead, the server performs the verification by looking at the signed tokens.
The JWTs carry a special message authentication code (MAC) that confirms the authenticity of their contained claims.
JWTs are either signed using a special HMAC algorithm or a private and public key pair. This key pair ensures the protection of the claim or information.
Token Expiration
When a user receives an access token, it comes with details about a refresh token and an expiration time. For example:
{
"access_token": "AYjcyMzY3ZDhiNmJkNTY",
"refresh_token": "RjY2NjM5NzA2OWJjuE7c",
"token_type": "bearer",
"expires": 3600
}
The refresh token means that the access token has a defined expiration period, and the user will get a new one.
The "expires" number is the number of seconds for which the access token will be valid. Usually, it's the service that specifies the token expiration period.
If you're using a JSON-based API and the token has expired already, you will likely get a JSON error response with the invalid_token
error.
HTTP/1.1 401 Unauthorized
WWW-Authenticate: Bearer error="invalid_token"
error_description="The access token expired"
Content-type: application/JSON
{
"error": "invalid_token",
"error"_description": "The access token expired"
}
When your code identifies this error, it initiates a request to the token endpoint by using the refresh token it received before and will obtain a new access token that it can use to make the original request again.
JSON Web Token Structure
JWTs consist of three parts: header, payload, and signature. A dot (.) is used between each part to differentiate it from the other. The structure looks like this: Header.Payload.Signature. Let's break down each of them in detail.
Token Header
The header is divided into two parts: the token's type (JWT) and the hashing or signed algorithm used (HMAC SHA256 or RSA).
The JSON is Base64Url encoded to form the header.
Token Payload
The payload is the part where the actual claim or information is stored. Claims are divided into three types: registered, public, and private.
Registered Claims
These are a set of claims which are pre-defined and not mandatory, but they are recommended to provide useful claims, including:
- exp (expiration time)
- iss (issuer)
- sub (subject)
- aud (audience)
Since JWTs need to be compact, the size of a registered claim is only three characters long.
Public Claims
The users can define public claims and are open for public consumption. But these claims can cause collisions with other names. To avoid such name clashes, the JWT user must define public claims in the IANA JSON Web Token Registry before using them.
Private Claims
Private claims are customized claims shared between two or more parties that agree on using them mutually. These claims are neither registered nor public.
Token Signature
The token signature ensures the authenticity of a message that it didn’t get changed before reaching its destination. The private key ensures the privacy of a token it is signed with. These keys can also verify the sender of the JWT.
A signature consists of:
- The encoded header
- The encoded payload
- A secret
- The header's algorithm (HMAC SHA256)
How Do JWTs Work?
A JSON Web Token is returned in authentication when the user succeeds in signing in with their credentials. It's recommended that users not keep tokens for an extended period as it can pose severe security issues.
Whenever the user intends to access a protected server, they need to send the JWT, generally in the Authorization header, with the help of the Bearer schema. The Bearer schema or Bearer authentication is an HTTP authentication scheme that includes security tokens called bearer tokens. The name "Bearer authentication" actually refers to "give access to the bearer of this token."
The Authorization Bearer header looks like this:
Authorization: Bearer <token>
In some cases, this step can be a stateless authorization method. The server's routes will evaluate whether the JWT is valid in the Authorization header. If the route identifies a valid JWT, the user will access the protected resources.
CORS is a browser mechanism that allows access to resources outside a specific domain. To ensure security, many browsers prohibit cross-origin HTTP requests. When the token is sent in the Authorization header, cross-origin resource sharing (CORS) is no longer a problem since it doesn't use user credentials, such as cookies, on requests.
How Can JWTs Be Used?
JWT should be used for API or user authentication and server-to-server or user authorization.
User Authentication
User authentication is the process that involves the verification of the user. It ensures that the user is the same person they claim to be.
At this step, the user needs to validate their credentials through providing ID or passwords, answering security questions, or using facial recognition. User authentication is generally performed before user authorization.
User Authorization
It is the process that involves the verification of the resources the user can or cannot access. This step comes after user authentication, where the user is granted access to certain resources based on policies and rules.
The Benefits of JWTs
The use of tokens, especially JWTs, has many benefits.
Increased User Security
JWTs ensure fool-proof user security. These tokens use a public and private key pair for a double layer of protection and provide users with an improved authentication experience.
Moreover, both signed and encrypted JWTs ensure the integrity of the claims. They also carry a special MAC that makes sure that the claim or information isn't changed before reaching the destination.
Compact and Self-Contained
The JWT is a compact and self-contained token that has all the authentication information, expiration time, and other pre-defined claims signed digitally.
Security Risks and Security Issues With JWTs
The JSON Web Token is a secure way of transmitting information, but this doesn't mean that it won't have any security risks. Some security risks and issues with JWTs are the following.
Problems in Verifying the Signature
Most JWT libraries offer one method for the decoding and the other for the verification:
- decode(): It doesn't verify the signature but decodes the token from base64url encoding.
- verify(): It verifies the signature as well as decodes the token.
Often, developers mix up these two methods, and the signature doesn't get verified. This means the application can now accept any type of token in a valid format. In addition, some developers may also forget to enable signature verification. Such things can make the token vulnerable to security breaches.
Allowing the None Algorithm
JWTs accept different types of algorithms, such as RSA or HMAC, to create a signature. However, it also allows the None algorithm, which refers to an un-signed token.
When JWTs permit this algorithm, the user can easily pass through the signature checking by changing an existing algorithm to None. When this happens, the hacker can smoothly replace the valid algorithm and remove the signature.
JSON Web Tokens vs. Simple Web Tokens (SWTs)
When JSON is encoded, its size becomes way smaller than XML. This makes JWTs more compact and precise than security assertion markup language (SAML) tokens and SWTs. Apart from that, the compact size of JWTs also helps them pass in HTML and HTTP environments easily.
When it comes to security, SWTs only use a single key. JWTs use a public and private key pair, primarily an X.509 certificate, for improved authentication. In addition, signing JSON is way simpler than signing XML. JSON parsers are also used more in most programming languages as they have a document-to-object mapping, which others fail to offer. Usage-wise, JWTs can be easily used on multiple devices, such as mobiles and laptops.
All of this makes JSON web tokens easier to work with across various platforms.
JWT-Based Authentication Summarized
All in all, JWT-based authentication is an excellent and secure way of transmitting information between multiple entities. The signatures on JWTs ensure the protection of the claims or information. All the user has to do is remember the correct key. Apart from that, JWTs are easier to understand and more compact than other web tokens like SAML or SWTs.
JWTs are also used at the internet scale, making them easier to process on a wide range of devices. However, it also poses some security vulnerabilities, like allowing the None algorithm and passing an unsigned and unverified token. If you want a better option, you can opt for a session management software that manages your session's lifecycle and authentication requests on its own.