Validating, Creating, and Styling React-Bootstrap Forms
- Category
- Guides
- Published
Create a complete login and sign-up flow with React Bootstrap forms, then learn how to use tools like Clerk to easily manage authentication and authorization.
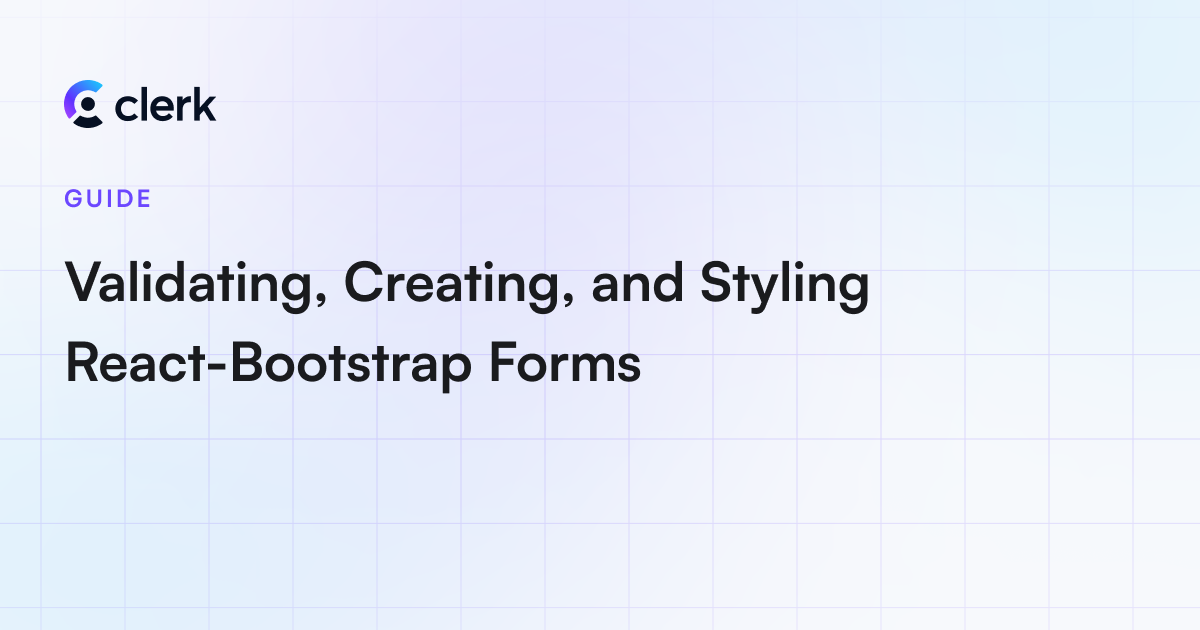
Building user interfaces works best by building small components and then combining and reusing them across your application. This ensures that different components in your application are not tightly coupled and encourages reusability. React.js, or React, is a free and open source JavaScript library that can help you do this.
However, creating a new page in React can be difficult, especially if you have a complex view with multiple components that must be rendered based on various conditions. This is where React-Bootstrap comes in.
React-Bootstrap is a frontend library providing an array of components and many other functionalities out of the box that you can directly import into your website instead of building them from scratch. One such component is bootstrap forms that you can use to easily create and design complex forms in React.
In this article, you'll learn about React-Bootstrap by creating a complete login and sign-up flow with bootstrap forms; you'll then see how to use tools like Clerk to further ease your life in terms of managing authentication and authorization flow.
Let's start with React-Bootstrap; the next section will talk about what React-Bootstrap forms are and how they can help you increase the quality of your application while saving you time and effort.
What Is React-Bootstrap?
React-Bootstrap is a frontend component library that provides pure React components. React-Bootstrap includes a plethora of ready-to-use components that you can import directly into your application. It's also very simple to make these components responsive, which would otherwise be a very time-consuming task.
The documentation for React-Bootstrap is also very well supported and simple to understand, allowing you to get started with a lower learning curve.
Why Use React-Bootstrap Forms?
There are several scenarios in which you might want to create forms for your website. Perhaps you need a sign-in/sign-up page for your customers to log in, an address page for an e-commerce app, or have some other use case where you want multiple inputs from your app's user. Even something as simple as adding a task to your to-do app requires a form.
Creating complex workflows in forms can be difficult. You must have an array of input types, such as text, password, and so on, as well as various validations based on input types and state attributes. This can eventually lead to hundreds of lines of code that are difficult to understand and debug.
This is where React-Bootstrap forms shine. With bootstrap forms, you can create a form with minimal code.
In the following sections, you'll learn how to design and build a sign-in/sign-up page using bootstrap forms. Let's get started!
Implementation
In this section, you'll first see how to create a React project and then use React-Bootstrap to create your own sign-in/sign-up page.
Project Setup
Before you can start writing code, you must first install React, React-Bootstrap, and other dependencies in your project. If you already have these installed, you can skip this section.
Installing npm and Node.js
Run the commands node —version
and npm —version
to see if Node.js and npm are already installed. If it says command not found
for either command, proceed to the next step.
The first thing you need to do is download a Node.js installer for your system. As recommended, download the LTS version. Install and run the software. This will automatically install Node.js and npm for you.
Once you're done installing, run the above commands once more to verify that both npm and Node.js have been correctly installed.
Setting Up a React Project
Once you have npm installed, it's easy to create a React project. Just run the command npx create-react-app <projectname>
. This example will use "bootstraplogin" as the project name.
Once the project is created, you need to install React-Bootstrap. For that, first run cd bootstraplogin
and then run npm install react-bootstrap bootstrap
. This will install React-Bootstrap and bootstrap to your project and add them as dependencies in your package.json file.
To ensure that everything is working as it should, run the command npm start
and visit http://localhost:3000
to ensure that the React default page is getting loaded like this.
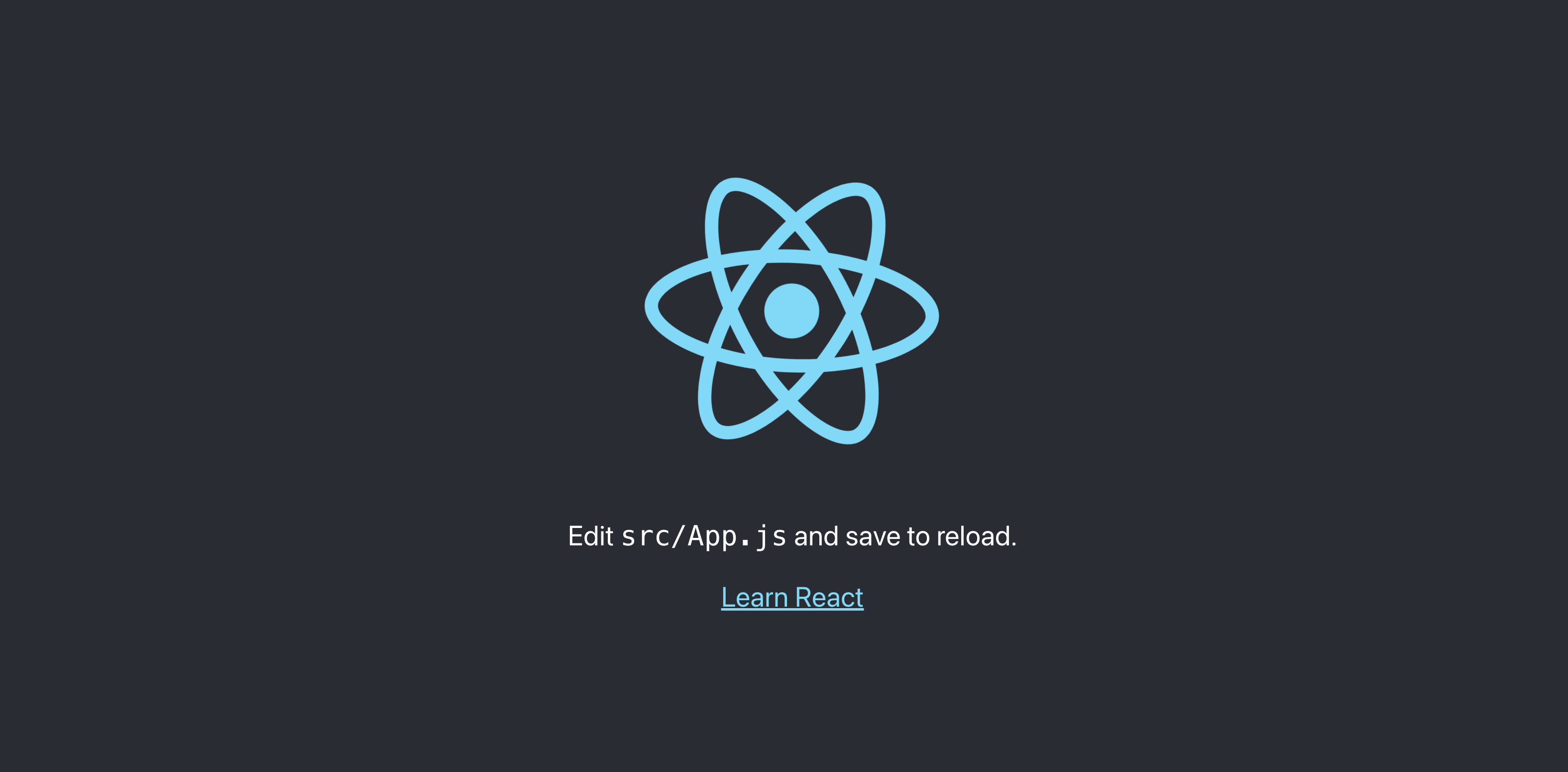
If this page is loading correctly, your setup is complete.
Creating a Form Using React-Bootstrap
In the section below, you'll get to grips with form creation using React-Bootstrap. You'll create and style a basic form in which users are asked to provide their email and password in order to sign in.
React-Bootstrap Form Basics
Before you dive into implementing your login flow, let's get the basics of bootstrap forms right.
Initially, your App.js file, which you can find in the src
folder of the project, will look something like this:
import logo from './logo.svg'
import './App.css'
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
)
}
export default App
You will now update your App.js to create a basic form page as described below.
The first thing you need to do is import the React-Bootstrap CSS. For that, add import 'bootstrap/dist/css/bootstrap.min.css';
to your index.js file.
Once that's done, update your App.js file to use bootstrap forms as below:
import React from 'react'
import Button from 'react-bootstrap/Button'
import Form from 'react-bootstrap/Form'
function App() {
return (
<Form>
<Form.Group className="mb-3" controlId="formBasicEmail">
<Form.Label>Email address</Form.Label>
<Form.Control type="email" placeholder="Enter email" />
<Form.Text className="text-muted">We'll never share your email with anyone else.</Form.Text>
</Form.Group>
<Form.Group className="mb-3" controlId="formBasicPassword">
<Form.Label>Password</Form.Label>
<Form.Control type="password" placeholder="Password" />
</Form.Group>
<Form.Group className="mb-3" controlId="formBasicCheckbox">
<Form.Check type="checkbox" label="Check me out" />
</Form.Group>
<Button variant="primary" type="submit">
Submit
</Button>
</Form>
)
}
export default App
The UI should now look like this.
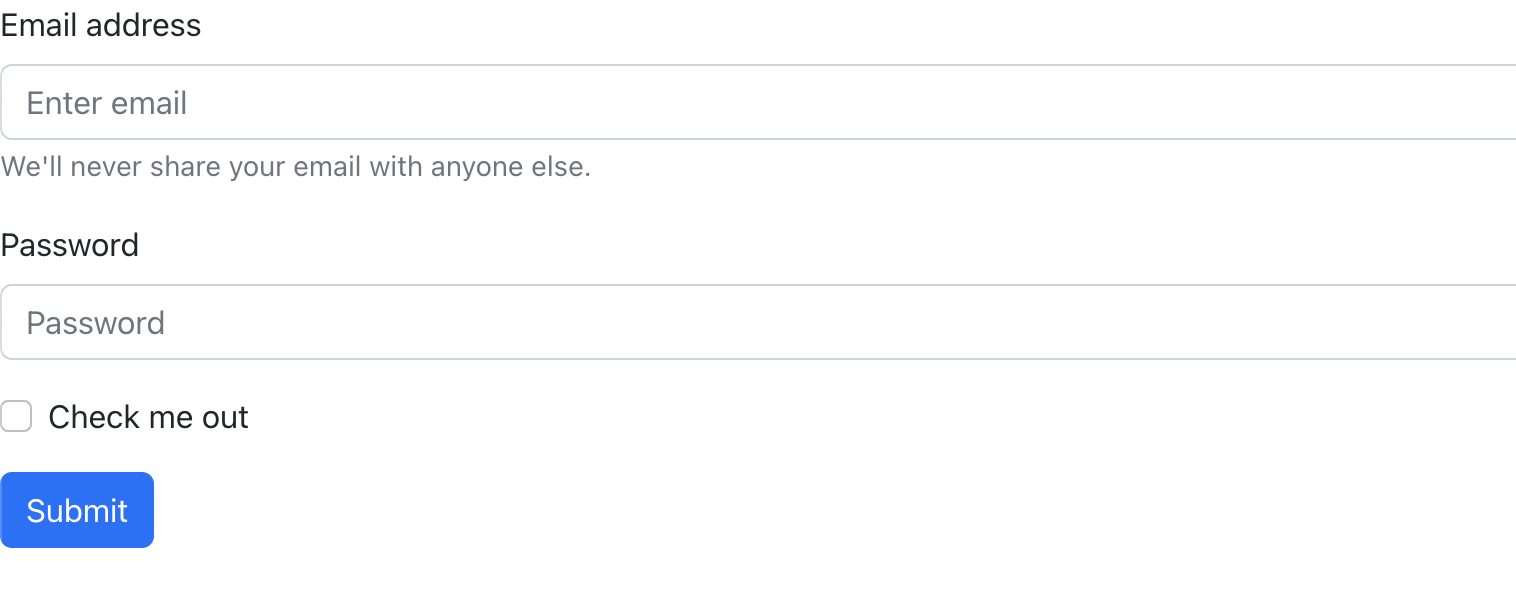
The first thing to notice in the above example is that you're importing Form
from react-bootstrap/Form
.
<Form>
is the top-level API provided by React-Bootstrap, which wraps all the functionality required to build forms.
The <Form.Control>
component renders the input component with bootstrap styling. Forms support different types of inputs like plaintext, email, password, file, or even color as input.
After that, you have <Form.Group>
, which creates a section inside the form view by wrapping the <Form.Control>
with its own styling and validations.
There's also <Form.Label>
, which works as the title for the form group, and <Form.Text>
, where you can add additional text.
Styling with React-Bootstrap
React-Bootstrap provides two main features for users to easily build responsive and well-designed web pages.
-
The grid system: Bootstrap's grid system uses a series of containers, rows, and columns to lay out and align content. It's built with Flexbox and is fully responsive. The grid also offers the following:
- Containers: Containers provide a means to center and horizontally pad your site's contents. Use the container feature for a responsive pixel width. You can use containers as the wrapper for your component to correctly align it.
- Row: Row is used to align elements in rows one after another.
- Column: Column is used to align elements as columns inside a row.
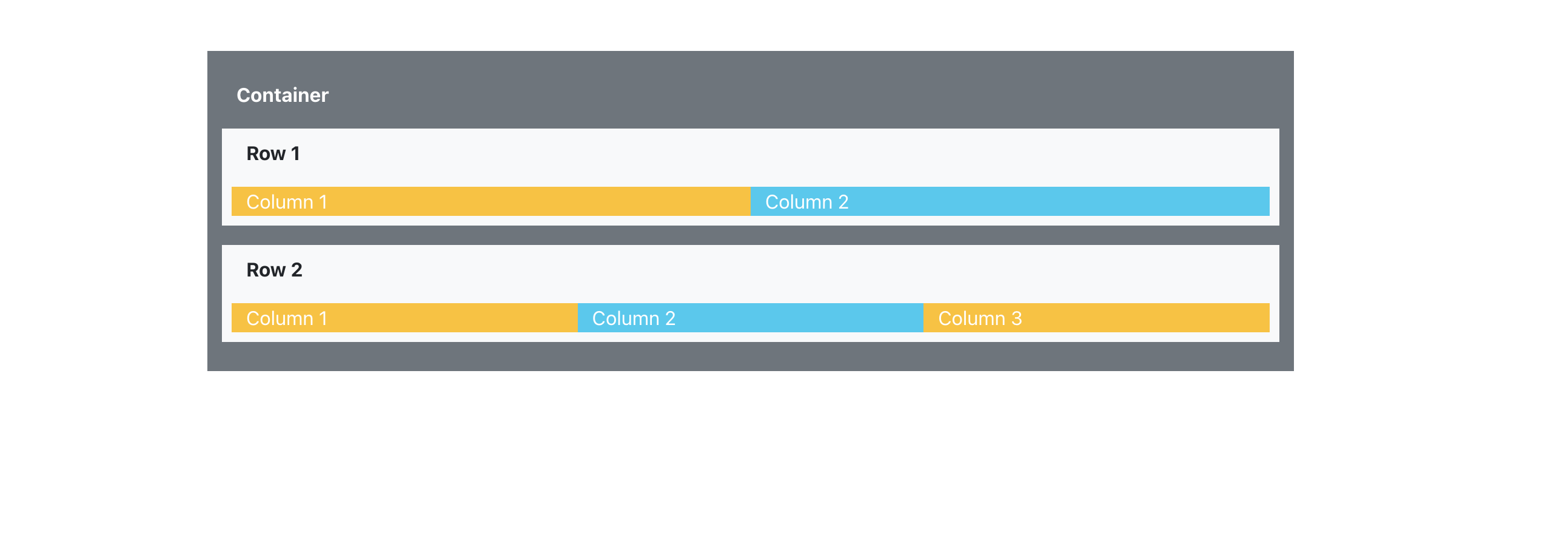
- CSS utilities: Bootstrap provides a SASS-based utility class to add CSS to your components. Using the utility API, you can easily add various CSS properties like border, color, background, etc. You can add these utilities with any component by adding
className
to your component.
For example, <div className="mb-3 mt-4"/>
provides a bottom margin of 3 pixels and a top margin of 4 pixels for the div.
Now that you've covered the fundamentals, let's create a sign-in/sign-up page for your website. You can find the entire code in this GitHub repo.
Sign-In Page
The first thing to do is create the SignIn.js file in your src
folder and add the below code:
import React from 'react'
import { Col, Button, Row, Container, Card, Form } from 'react-bootstrap'
export default function Login() {
return (
<Container>
<Row className="vh-100 d-flex justify-content-center align-items-center">
<Col md={8} lg={6} xs={12}>
<div className="border-3 border-primary border"></div>
<Card className="shadow">
<Card.Body>
<div className="mb-3 mt-4">
<h2 className="fw-bold text-uppercase mb-2">Brand</h2>
<p className="mb-5">Please enter your login and password!</p>
<Form className="mb-3">
<Form.Group className="mb-3" controlId="formBasicEmail">
<Form.Label className="text-center">Email address</Form.Label>
<Form.Control type="email" placeholder="Enter email" />
</Form.Group>
<Form.Group className="mb-3" controlId="formBasicPassword">
<Form.Label>Password</Form.Label>
<Form.Control type="password" placeholder="Password" />
</Form.Group>
<div className="mb-3">
<p className="small">
<a className="text-primary" href="#!">
Forgot password?
</a>
</p>
</div>
<div className="d-grid">
<Button variant="primary" type="submit">
Login
</Button>
</div>
</Form>
<div className="mt-3">
<p className="mb-0 text-center">
Don't have an account?{' '}
<a href="{''}" className="text-primary fw-bold">
Sign In
</a>
</p>
</div>
</div>
</Card.Body>
</Card>
</Col>
</Row>
</Container>
)
}
Now, let's see exactly what's happening in the above example.
- You use a
<Container>
to wrap the component. Containers provide an easy means to center and horizontally pad the content you put inside it. - Then, you use a
<Row>
and a<Col>
element. The former is used to add CSS to the component to orient the entire screen horizontally and align the internal component to center; the latter is used to add breakpoints to ensure a responsive view across any screen size. - Next,
<div>
adds a blue horizontal line to the view for which the div is styled using the border utility provided by React-Bootstrap. - Next, you have
<Card>
and<Card.Body>
. Card is a basic container with inherent styling like a rounded border and some padding. Inside the card, you can have a header, footer, and body. In the example, you only use Card.Body. - Then there's a
<div>
with className values asmb-3
andmt-4
, which provide a bottom margin of 3 pixels and a top margin of 4 pixels to the div. - After that, you add an
<h2>
tag for the heading in the view.fw-bold
sets font weight as bold,text-uppercase
makes the text uppercase, whilemb-2
is again setting 2 pixels as the bottom margin. - After that, you use the
<p>
tag to add text. - Next, you want to add inputs for the user to add username and password. For this, you use
<Form>
. - In code, there are two groups in
<Form>
: one for the username and the other for the password using<Form.Group>
.ControlId
here works as a unique id for the FormGroup. - Then, you add
<Form.Label>
to add a heading for the input. <Form.Control>
converts to the actual HTML input element in the view.type
is given asemail
, which checks for a valid email address, andplaceholder
is added, which will be shown when the user has not yet provided input.- You then repeat steps 10 and 11 but this time for the password. Adding
type
asPassword
forForm.Control
also ensures that the input is not visible but is added as a**
. - After that, you add a
<div>
, wrapping a clickable link and ap
tag inside it; thep
tag hasclassName
"small" and "text-primary", which sets size and color for the inside text respectively. - An anchor tag is used to make the text clickable. You can also add an actual link that provides "Forgot password" functionality by replacing
#
with that link. - After that, there's another
<div>
wrapping a clickable button. If you want to find out more, React-Bootstrap provides an API for buttons. - Finally, you add another
<div>
with a link that once clicked should open a sign-up page for the user.
Your sign-in page should look like below.
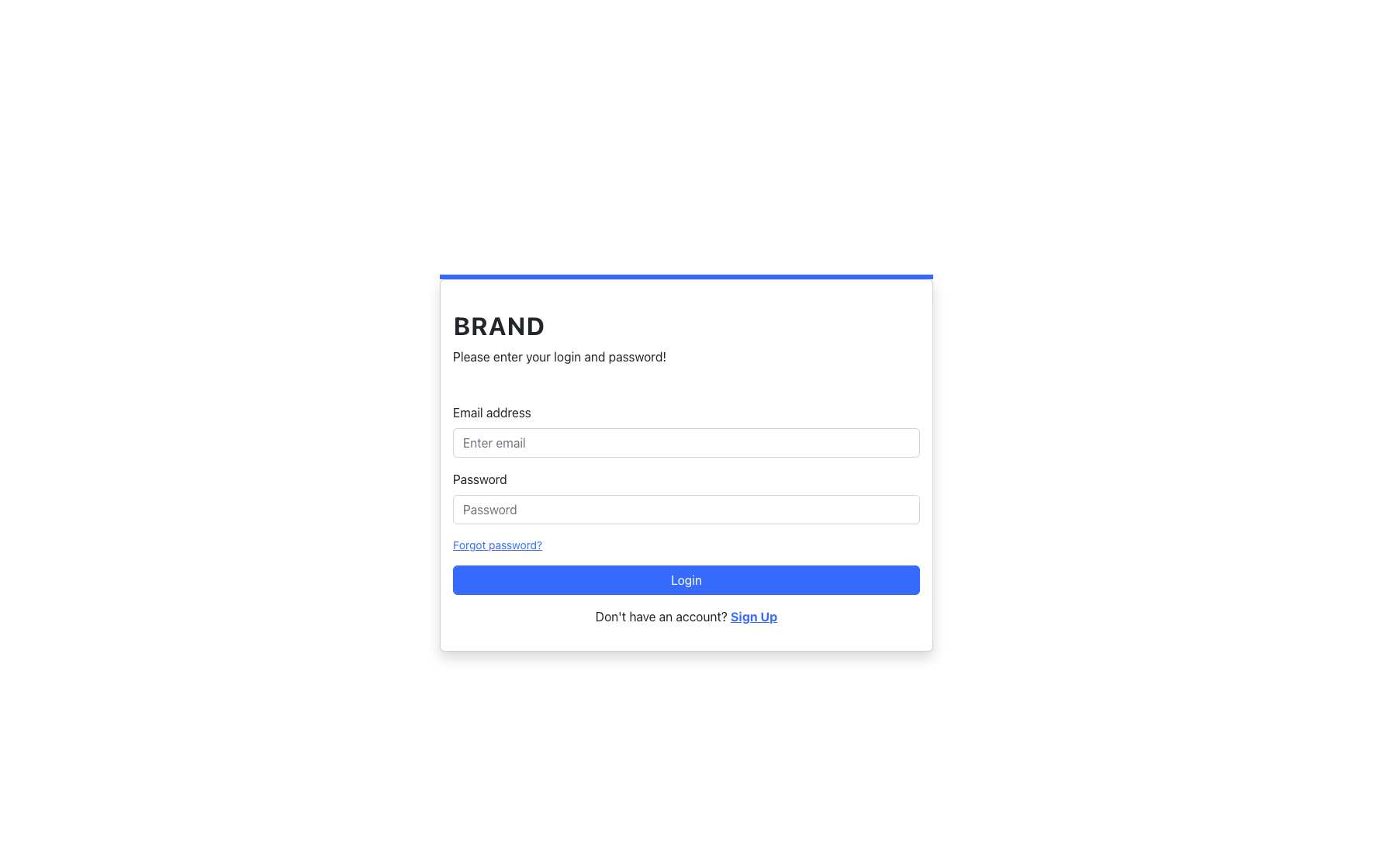
Sign-Up Page
The sign-up page has similar components to the sign-in page but requires some extra details, which you'll see below.
First, you need to create the SignUp.js file in your src
folder and add the below code:
import React from 'react'
import { InputGroup, Col, Button, Row, Container, Card, Form } from 'react-bootstrap'
export default function SignUp() {
return (
<Container>
<Row className="vh-100 d-flex justify-content-center align-items-center">
<Col md={10} lg={8} xs={12}>
<div className="border-3 border-primary border"></div>
<Card className="shadow">
<Card.Body>
<div className="mb-3 mt-4">
<h2 className="fw-bold text-uppercase mb-2">Brand</h2>
<p className="mb-5">Please enter your details to join us!</p>
<Form>
<Row className="mb-3">
<Form.Group as={Col} className="mb-3" controlId="formFullName">
<Form.Label className="text-center">Your full name</Form.Label>
<Form.Control type="text" placeholder="Enter name" />
</Form.Group>
<Form.Group as={Col} className="mb-3" controlId="formPhoneNumber">
<Form.Label>Phone Number</Form.Label>
<Form.Control type="number" placeholder="Enter phone number" />
</Form.Group>
</Row>
<Row className="mb-3">
<Form.Group as={Col} className="mb-3" controlId="formUsername">
<Form.Label className="text-center">Email address</Form.Label>
<InputGroup>
<Form.Control type="email" placeholder="Enter username" />
<InputGroup.Text className="text-primary">@brand.com</InputGroup.Text>
</InputGroup>
</Form.Group>
<Form.Group as={Col} className="mb-3" controlId="formBasicPassword">
<Form.Label>Password</Form.Label>
<Form.Control type="password" placeholder="Password" />
</Form.Group>
</Row>
<div className="d-grid">
<Button variant="primary" type="submit">
Sign Up
</Button>
</div>
</Form>
<div className="mt-3">
<p className="mb-0 text-center">
Already have an account?{' '}
<a href="{''}" className="text-primary fw-bold">
Sign In
</a>
</p>
</div>
</div>
</Card.Body>
</Card>
</Col>
</Row>
</Container>
)
}
Let's see what each line of code does.
- Similarly to the sign-in page, you create a container, then add a row and column to it.
- You again have a div representing the horizontal line just for the view.
- Then you once more add
<Card>
, including<Card.Body>
, which captures the header text, and then the<Form>
. - Now you have a
<Row>
, which was not the case in the sign-in page. Row has two<Form.Group>
s: one for a name and another for a phone number. This is so that these two form groups can be rendered in a single row. Along with adding<Row>
, you also have to addas={Col}
to the<Form.Group>
to align these two form groups in a single row. - You again have a
<Row>
, which includes two form groups. These form groups are for users to add their username and password. - In the username form group,
<InputGroup>
is added. InputGroup easily extends the Form.Control component by adding text, buttons, or button groups on either side of the input. - In the above code,
@brand.com
is added as<InputGroup>
text, which signifies that the email address for the user will be "username@brand.com". - After that, much like with the sign-in page, you have a clickable button for signing up and a link to move to the sign-in page.
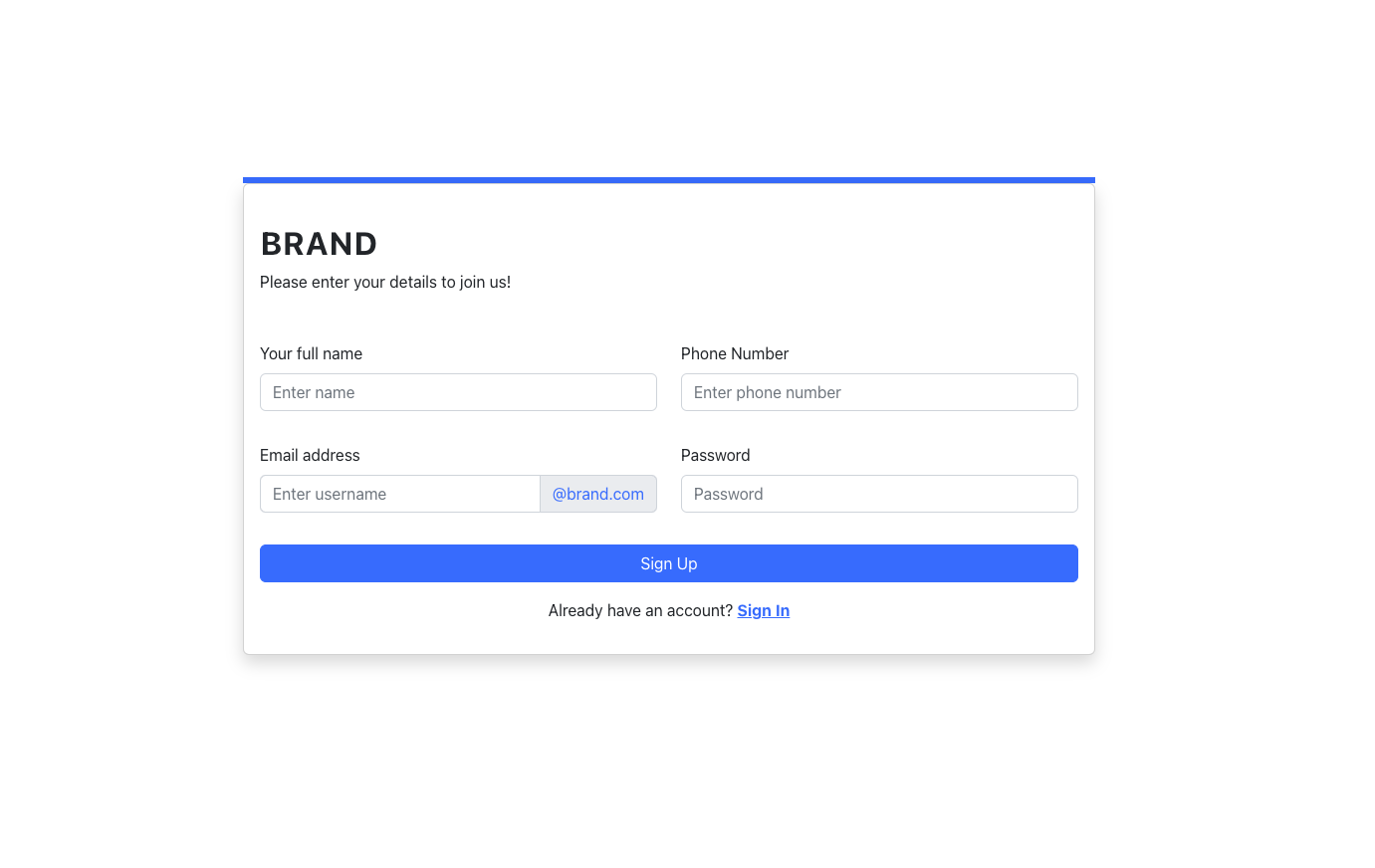
What's Next?
You saw above how easy it is to create your own sign-in and sign-up page using Bootstrap; however, there are aspects that Bootstrap doesn't automatically take care of, which are essential in creating a secure and functional website. For example, along with asking the user to upload their information, you also need to store that information in your database and validate the username and password when the user tries to log in.
There are various other features like authorization, social logins, and multifactor authentication, which are very complex workflows in themselves where you must ensure that your system's security is never compromised. This means that as the product owner, you must keep up with advancements to ensure a secure product.
In light of these considerations, it might be a good idea for you to delegate the sign-in/sign-up functionality to a SaaS platform like Clerk that takes care of all these complex tasks for you.
Using Clerk to Create More Secure Login Forms
Clerk is a tool that makes it simple for you to integrate authentication and authorization functionality with your web and mobile apps without having to worry about low-level security details or even the design of the sign-in/sign-up page.
Creating a Login Form Using Clerk
Before we get into integrating Clerk into your application, you'll have to create an account. Sign up with Clerk and you'll be shown the below page.
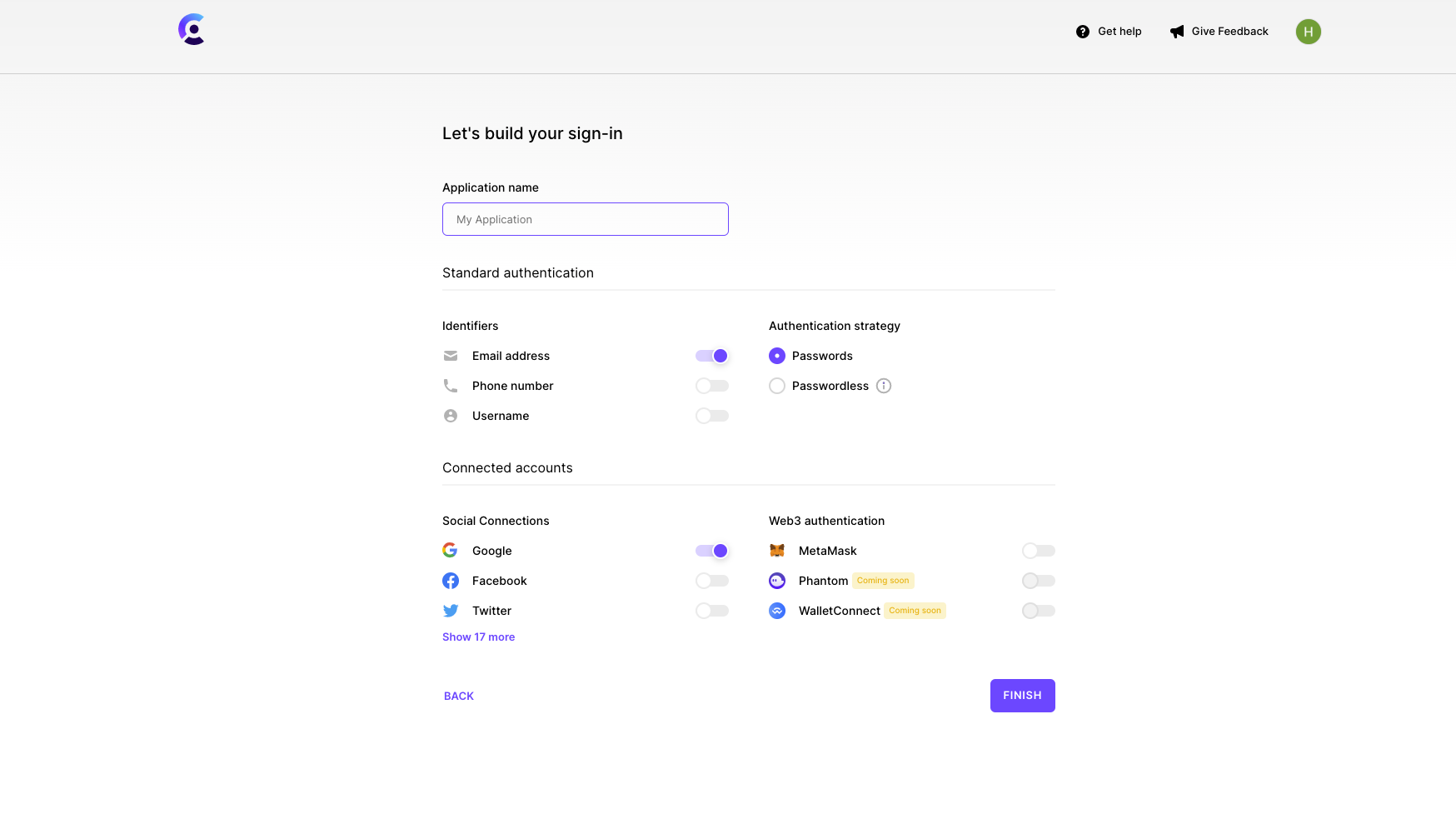
Let's go section by section. First, you need your application name. You can add more applications later, so make sure the name is specific to the project you're working on.
After that, you need to specify how you want to authenticate your users. You can choose multiple options based on your requirements. You can also mention the social media platforms you want to use for authentication.
Once you're done, click Submit and you'll be directed to your application page. Just click Try it now and it will redirect you to your sign-in/sign-up page.
Integrating Clerk with Your Application
Integrating Clerk with your React application is a simple process. All you have to do is follow the instructions in this article. Let's set it up step by step below.
Set Up Clerk in Your Project
- Go to your project repository in the command line, if you're not already there, using
cd <path_to_your_project>
. - Install Clerk React SDK using
npm install @clerk/clerk-react
. - Create a file to store
env
variables using thetouch .env.local
command. - Run
echo "REACT_APP_CLERK_FRONTEND_API=clerk.sharp.mustang-37.lcl.dev" > .env.local
. This will addREACT_APP_CLERK_FRONTEND_API
as anenv
variable in your file.
And that's all you need to integrate with Clerk. It's fairly easy, right? Now let's go and make the required code changes to integrate your application with Clerk.
Code Changes
Add the below code to the App.js file in your src
folder:
import React from 'react'
import './App.css'
import {
ClerkProvider,
SignedIn,
SignedOut,
UserButton,
useUser,
RedirectToSignIn,
} from '@clerk/clerk-react'
const frontendApi = process.env.REACT_APP_CLERK_FRONTEND_API
function App() {
return (
// Wrap your entire app with ClerkProvider
// Don't forget to pass the frontendApi prop
<ClerkProvider frontendApi={frontendApi}>
<SignedIn>
<Hello />
</SignedIn>
<SignedOut>
<RedirectToSignIn />
</SignedOut>
</ClerkProvider>
)
}
function Hello() {
// Get the user's first name
const { user } = useUser()
return (
<div className="App-header">
{/* Mount the UserButton component */}
<UserButton />
{user ? <h1>Hello, {user.firstName}!</h1> : null}
</div>
)
}
export default App
Now let's see what the above code is doing:
- You import the required dependencies from the Clerk module.
frontendApi
tells the Clerk framework which API endpoint to hit to get the user-related information.- The
<ClerkProvider>
component wraps your React application to provide active session and user context. It must always be added to the entrypoint of your React application. - The
<SignedIn>
component offers authentication checks for your application. Any child components wrapped by aSignedIn
component will be rendered only if there's a user signed into an active session in your application. For example, when you want the cart and profile page to be visible only to logged-in users, you can use<SignedIn>
. - Similarly, any child nodes wrapped by a
<SignedOut>
component will be rendered only if the user is not yet signed in to your application. - In the
Hello
component, theuseUser
hook gets the information regarding the logged-in user. The<UserButton>
provides you with a clickable button. - In your application, if the user is signed in, it will show the
<Hello />
component, which shows user information and a<UserButton>
. - If the user is signed out, you're just redirecting the app to show the sign-in page using
<RedirectToSignIn>
.
The signed-out view looks like the following.
And here's the signed-in view.
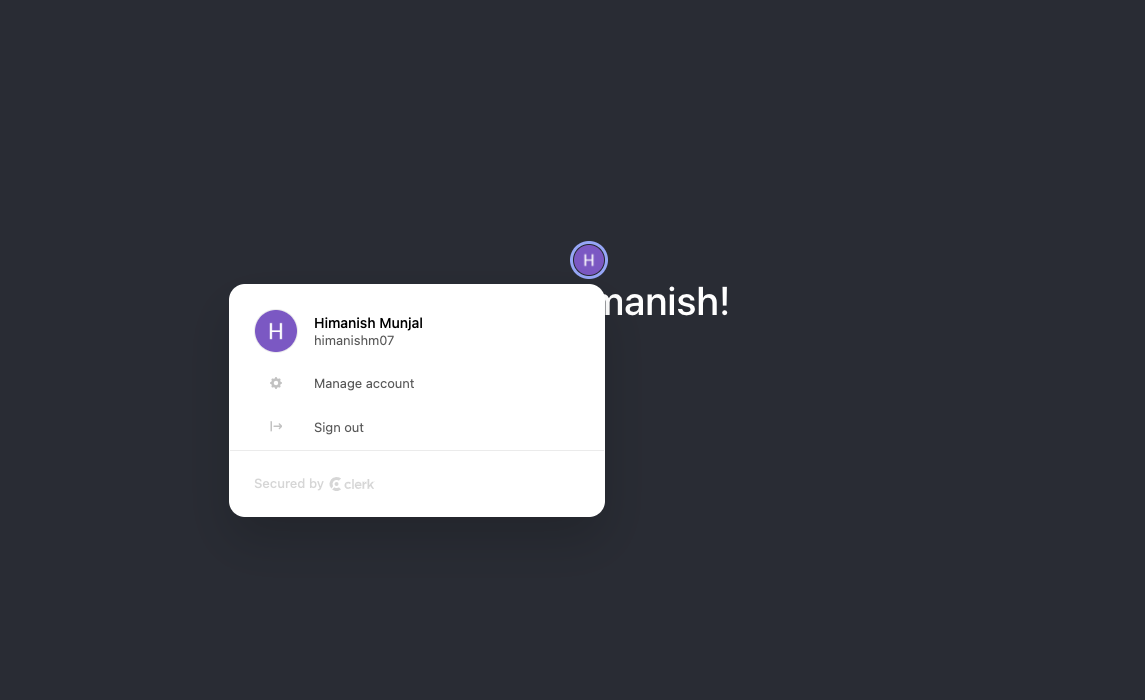
Clerk.com vs. React-Bootstrap
Now that you understand how Clerk works and what features it provides, let's discuss the benefits it offers over implementing your own login flow using React-Bootstrap.
- Customized UI: With Clerk, you can create your sign-in/sign-up pages with just a few clicks. You don't need to worry about creating different components, styling them, and handling their state. Just import the ClerkProvider and you're done.
- Offloading complexity of authentication and authorization: As discussed above, implementing authentication and authorization for your application is a very complex task. Clerk takes care of this complexity for you.
- Advanced functionality out of the box: Along with authentication and authorization, Clerk provides various other functionalities like social media links and single sign-on (SSO), multifactor authentication, phone-number- and email-based login, and many other features.
- Seamless integration with different projects: With Clerk, you can integrate your login module with different frontend applications, be it mobile apps, web pages, etc. If you're migrating from one JS framework to another—for example, from React.js to Next.js—you just need to integrate with your new project rather than write all the code again.
- Better control and monitoring: Clerk also provides you with the metadata information like registered users, registration date, and last sign in. You can also integrate with Google Analytics and Firebase for analyzing data.
Conclusion
In this article, you learned how to create your sign-in and sign-up page, first using React-Bootstrap and then recreating it using Clerk. You also learned various benefits of React-Bootstrap and Clerk and how the latter enables you to completely offload the complex authentication and authorization flow.
Clerk helps you in building your sign-in and sign-up page just with a few clicks. It provides an array of functionalities like social sign in, SSO, multifactor authentication, user information, session management, etc. By doing so, it helps you focus on solving your business problems without worrying about the security of your application.