Appearance
prop
Customizing the appearance of Clerk components is a powerful way to make your application look and feel unique. Clerk provides a way to customize the appearance of its components using the appearance
prop.
The appearance
prop can be applied to <ClerkProvider>
to share styles across every component, or individually to any of the Clerk components.
This applies to all of the React-based packages, like , as well as .
Properties
The appearance
prop accepts the following properties:
- Name
baseTheme?
- Type
BaseTheme | BaseTheme[]
- Description
A theme used as the base theme for the components. For more information, see Themes.
- Name
layout?
- Type
Layout
- Description
Configuration options that affect the layout of the components, allowing customizations that are hard to implement with just CSS. For more information, see Layout.
- Name
variables?
- Type
Variables
- Description
General theme overrides. This styles will be merged with our base theme. Can override global styles like colors, fonts, etc. For more information, see Variables.
- Name
elements?
- Type
Elements
- Description
Fine-grained theme overrides. Useful when you want to style specific elements or elements that are under a specific state. For more information, see the Customize elements of a Clerk component section.
- Name
captcha?
- Type
Captcha
- Description
Configuration options that affect the appearance of the CAPTCHA widget. For more information, see the dedicated guide.
- Name
cssLayerName?
- Type
string
- Description
The name of the CSS layer for Clerk component styles. This is useful for advanced CSS customization, allowing you to control the cascade and prevent style conflicts by isolating Clerk's styles within a specific layer. For more information on CSS layers, see the MDN documentation on @layer.
Using a prebuilt theme
Clerk offers a set of prebuilt themes that can be used to quickly style Clerk components. See the Themes docs for more information.
Customize the layout
The layout
property is used to adjust the layout of the <SignIn/>
and <SignUp/>
components, as well as set important links to your support, terms, and privacy pages. See the Layout docs for more information.
Customize the base theme
The variables
property is used to adjust the general styles of a component's base theme, like colors, backgrounds, and typography. See the Variables docs for more information.
Customize elements of a Clerk component
If you want full control over the appearance of a Clerk component, you can target the underlying elements by using their CSS classes and then apply your own styles.
First, you need to identify the underlying element of the Clerk component you want to style. You can do this by inspecting the HTML of the component.
For example, if you want to style the primary button in a Clerk component, you can right-click on the primary button and select "Inspect" from the menu. This will open the browser's developer tools and highlight the element in the HTML, as shown in the following image:
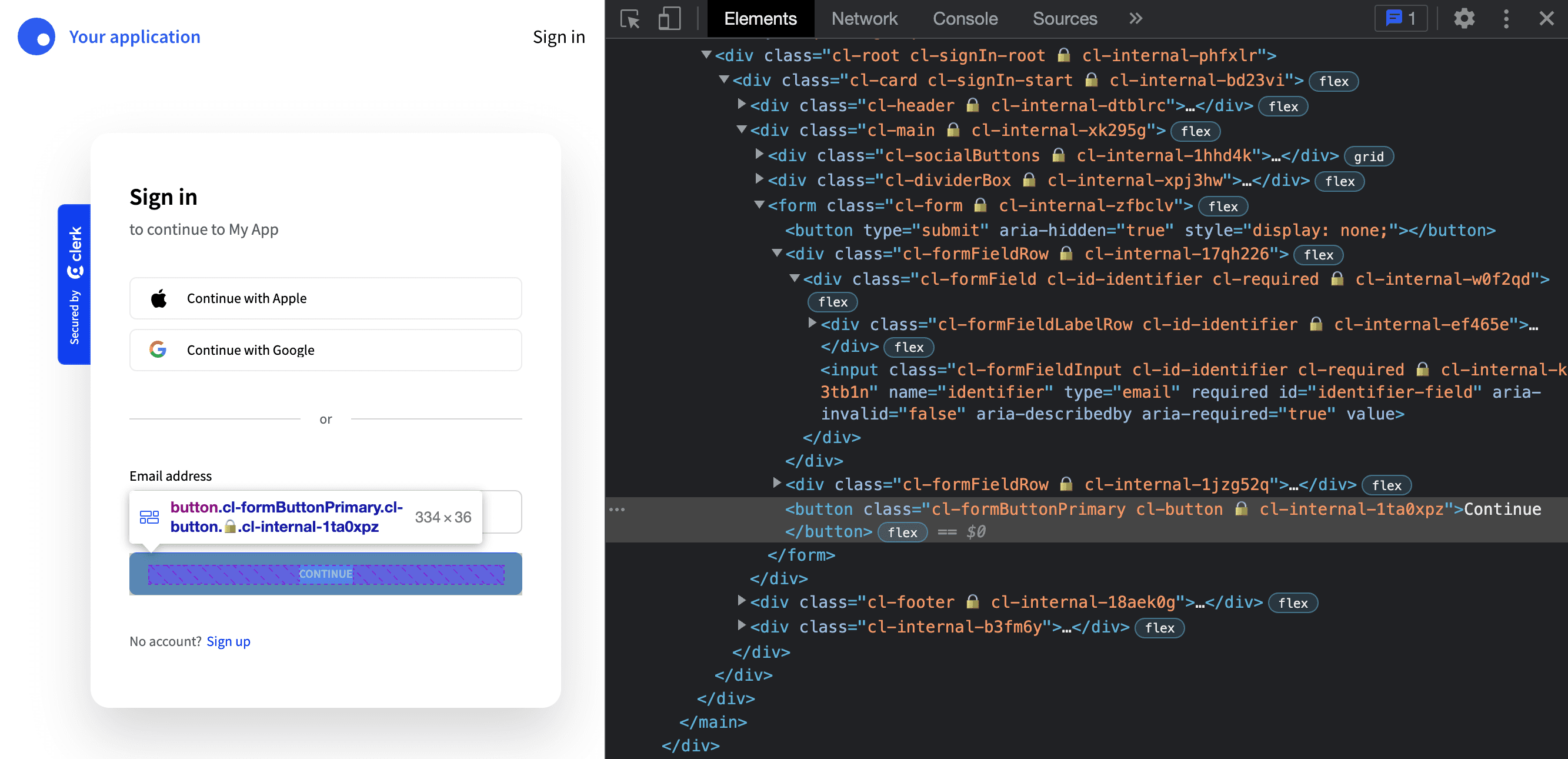
When you select an element that is part of a Clerk component, you'll notice a list of classes like so:
cl-formButtonPrimary cl-button 🔒️ cl-internal-1ta0xpz
Any of the classes listed before the lock icon (🔒️) are safe to rely on, such as cl-formButtonPrimary
or cl-button
from the previous example. You'll use these classes to target the necessary elements of the Clerk component.
Once you have identified the classes of the element you want to target, there are many ways to apply your custom styles depending on your preference:
Use global CSS to style Clerk components
You can style the elements of a Clerk component with global CSS.
For this example, say you want to style the primary button in a Clerk component. You inspect the primary button to find the classes that you can use to target the element:
cl-formButtonPrimary cl-button 🔒️ cl-internal-1ta0xpz
You can then create a global CSS file, use the classes you identified to target the primary button, and apply your custom styles. In this case, cl-formButtonPrimary
is the class you want to use because it's specific to the primary button:
.cl-formButtonPrimary {
font-size: 14px;
text-transform: none;
background-color: #611bbd;
}
.cl-formButtonPrimary:hover,
.cl-formButtonPrimary:focus,
.cl-formButtonPrimary:active {
background-color: #49247a;
}
Use custom CSS classes to style Clerk components
You can pass additional classes to Clerk component elements by using the elements
property on the appearance
prop.
For example, an element in a Clerk component will have classes that look something like this:
cl-formButtonPrimary cl-button 🔒️ cl-internal-1ta0xpz
Remove the cl-
prefix from a class and use it as the key for a new object in the elements
property. The value of this object should be the string of classes you want to apply to the element.
The following example shows how to style the primary button in a <SignIn />
component with custom CSS classes:
<SignIn
appearance={{
elements: {
formButtonPrimary: 'your-org-button org-red-button',
},
}}
/>
Use Tailwind classes to style Clerk components
To use Tailwind CSS v4, you must set the cssLayerName
property to ensure that Tailwind's utility styles are applied after Clerk's styles. It's recommended to add this to the <ClerkProvider>
that wraps your app so that it's applied to all Clerk components, as shown in the following example. The example names the layer clerk
but you can name it anything you want.
import { ClerkProvider } from '@clerk/nextjs'
export default function RootLayout({ children }: { children: React.ReactNode }) {
return (
<ClerkProvider
appearance={{
cssLayerName: 'clerk',
}}
>
<html lang="en">
<body>{children}</body>
</html>
</ClerkProvider>
)
}
@layer theme, base, clerk, components, utilities;
@import 'tailwindcss';
import { defineConfig } from 'astro/config'
import node from '@astrojs/node'
import clerk from '@clerk/astro'
import tailwind from '@tailwindcss/vite'
export default defineConfig({
integrations: [
clerk({
appearance: {
cssLayerName: 'clerk',
},
}),
],
output: 'server',
adapter: node({
mode: 'standalone',
}),
vite: {
plugins: [tailwind()],
},
})
@layer theme, base, clerk, components, utilities;
@import 'tailwindcss';
import { createApp } from 'vue'
import './style.css'
import App from './App.vue'
import { clerkPlugin } from '@clerk/vue'
const PUBLISHABLE_KEY = import.meta.env.VITE_CLERK_PUBLISHABLE_KEY
if (!PUBLISHABLE_KEY) {
throw new Error('Add your Clerk Publishable Key to the .env file')
}
const app = createApp(App)
app.use(clerkPlugin, {
appearance: {
cssLayerName: 'clerk',
},
publishableKey: PUBLISHABLE_KEY,
})
app.mount('#app')
@layer theme, base, clerk, components, utilities;
@import 'tailwindcss';
<script>
window.addEventListener('load', async function () {
await Clerk.load({
appearance: {
cssLayerName: 'clerk',
},
})
})
</script>
@layer theme, base, clerk, components, utilities;
@import 'tailwindcss';
Then, you can use Tailwind's classes to style the elements of the Clerk component. The following example shows how to use Tailwind classes to style the primary button in a <SignIn />
component:
<SignIn
appearance={{
elements: {
formButtonPrimary: 'bg-slate-500 hover:bg-slate-400 text-sm',
},
}}
/>
Use CSS modules to style Clerk components
CSS modules are a great way to scope your CSS to a specific component.
Create your module file and add the CSS you want to apply, as shown in the following example for the <SignIn />
component:
.primaryColor {
background-color: bisque;
color: black;
}
Then you can apply this by importing the file and using the classes whenever required:
import styles from '../styles/SignIn.module.css'
import { ClerkProvider, SignIn } from '@clerk/nextjs'
export default function RootLayout({ children }: { children: React.ReactNode }) {
return (
<ClerkProvider>
<html lang="en">
<body>
<SignIn
appearance={{
elements: {
formButtonPrimary: styles.primaryColor,
},
}}
/>
</body>
</html>
</ClerkProvider>
)
}
import styles from '../styles/SignIn.module.css'
import { ClerkProvider, SignIn } from '@clerk/nextjs'
import type { AppProps } from 'next/app'
function MyApp({ pageProps }: AppProps) {
return (
<ClerkProvider {...pageProps}>
<SignIn
appearance={{
elements: {
formButtonPrimary: styles.primaryColor,
},
}}
/>
</ClerkProvider>
)
}
export default MyApp
Use inline CSS objects to style Clerk components
You can style the elements of a Clerk component with inline CSS objects.
The following example shows how to style the primary button in a <SignIn />
component with an inline CSS object:
import styles from '../styles/SignIn.module.css'
import { ClerkProvider, SignIn } from '@clerk/nextjs'
export default function RootLayout({ children }: { children: React.ReactNode }) {
return (
<ClerkProvider>
<html lang="en">
<body>
<SignIn
appearance={{
elements: {
formButtonPrimary: {
fontSize: 14,
textTransform: 'none',
backgroundColor: '#611BBD',
'&:hover, &:focus, &:active': {
backgroundColor: '#49247A',
},
},
},
}}
/>
</body>
</html>
</ClerkProvider>
)
}
import { ClerkProvider, SignIn } from '@clerk/nextjs'
import type { AppProps } from 'next/app'
function MyApp({ pageProps }: AppProps) {
return (
<ClerkProvider {...pageProps}>
<SignIn
appearance={{
elements: {
formButtonPrimary: {
fontSize: 14,
textTransform: 'none',
backgroundColor: '#611BBD',
'&:hover, &:focus, &:active': {
backgroundColor: '#49247A',
},
},
},
}}
/>
</ClerkProvider>
)
}
export default MyApp
Next steps
Here are a few resources you can utilize to customize your Clerk components further:
Localization
Learn how to localize your Clerk components.
prebuilt themes
Explore the prebuilt themes that you can use to quickly style your Clerk components.
Customize layouts
Learn how to change the layout and links of your Clerk components.
Feedback
Last updated on