<OrganizationSwitcher />
component
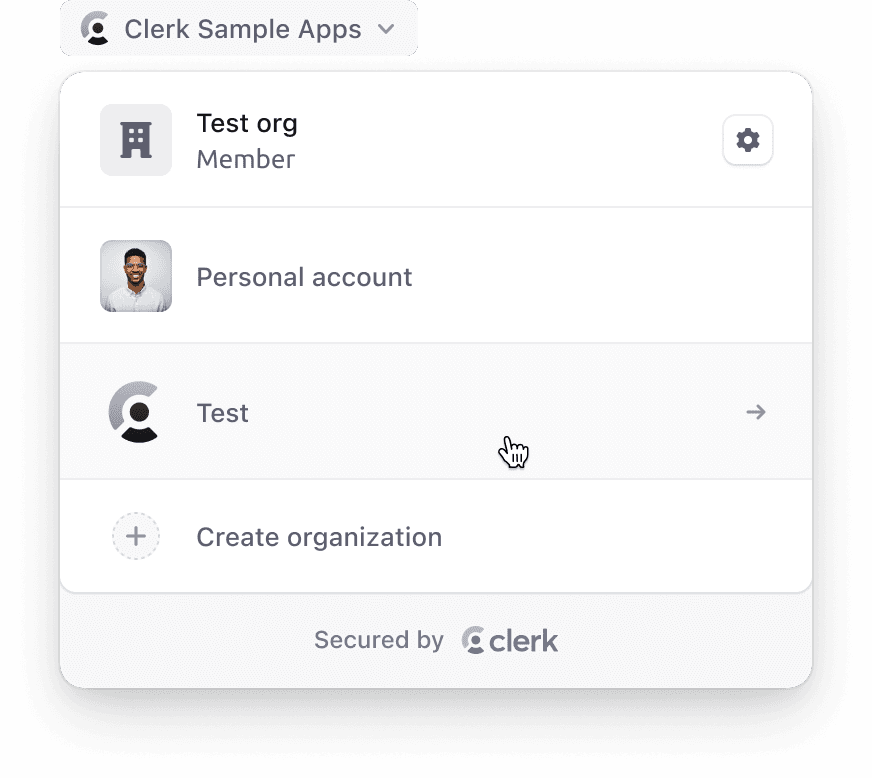
The <OrganizationSwitcher />
component allows a user to switch between their account types - their personal account and their joined organizations. This component is useful for applications that have a multi-tenant architecture, where users can be part of multiple organizations. It handles all organization-related flows, including full organization management for admins. Learn more about organizations.
If you would like to learn how to hide a user's personal account in order to enforce an organization-centric application, see the dedicated guide.
Properties
The <OrganizationSwitcher />
component accepts the following properties, all of which are optional:
- Name
afterCreateOrganizationUrl
- Type
string
- Description
The full URL or path to navigate to after creating a new organization.
- Name
afterLeaveOrganizationUrl
- Type
string
- Description
The full URL or path to navigate to after the user leaves the currently active organization.
- Name
afterSelectOrganizationUrl
- Type
string
- Description
The full URL or path to navigate to after a successful organization switch.
- Name
appearance
- Type
Appearance | undefined
- Description
Optional object to style your components. Will only affect Clerk components and not Account Portal pages.
- Name
createOrganizationMode
- Type
'modal' | 'navigation'
- Description
A boolean that controls whether clicking the "Create organization" button will cause the
<CreateOrganization />
component to open as a modal, or if the browser will navigate to thecreateOrganizationUrl
where<CreateOrganization />
is mounted as a page. Defaults to:'modal'
.
- Name
createOrganizationUrl
- Type
string
- Description
The full URL or path where the
<CreateOrganization />
]createorg-ref component is mounted.
- Name
defaultOpen
- Type
boolean
- Description
A boolean that controls the default state of the
<OrganizationSwitcher />
component.
- Name
fallback?
- Type
ReactNode
- Description
An optional element to be rendered while the component is mounting.
- Name
hidePersonal
- Type
boolean
- Description
A boolean that controls whether
<OrganizationSwitcher />
will include the user's personal account in the organization list. Setting this totrue
will hide the personal account option, and users will only be able to switch between organizations. Defaults tofalse
.
- Name
hideSlug
- Type
boolean
- Description
A boolean that controls whether the optional slug field in the organization creation screen is hidden.
- Name
organizationProfileMode
- Type
'modal' | 'navigation'
- Description
A boolean that controls whether clicking the Manage organization button will cause the
<OrganizationProfile />
component to open as a modal, or if the browser will navigate to theorganizationProfileUrl
where<OrganizationProfile />
is mounted as a page. Defaults to:'modal'
.
- Name
organizationProfileProps
- Type
object
- Description
Specify options for the underlying
<OrganizationProfile />
component. For example:{appearance: {...}}
- Name
organizationProfileUrl
- Type
string
- Description
The full URL or path where the
<OrganizationProfile />
component is mounted.
import { OrganizationSwitcher } from '@clerk/nextjs'
export default function OrganizationSwitcherPage() {
return <OrganizationSwitcher />
}
import { OrganizationSwitcher } from '@clerk/clerk-react'
export default function OrganizationSwitcherPage() {
return <OrganizationSwitcher />
}
---
import { OrganizationSwitcher } from '@clerk/astro/components'
---
<OrganizationSwitcher />
import { OrganizationSwitcher } from '@clerk/remix'
export default function OrganizationSwitcherPage() {
return <OrganizationSwitcher />
}
import { OrganizationSwitcher } from '@clerk/tanstack-react-start'
import { createFileRoute } from '@tanstack/react-router'
export const Route = createFileRoute('/organization-switcher')({
component: OrganizationSwitcherPage,
})
function OrganizationSwitcherPage() {
return <OrganizationSwitcher />
}
<script setup lang="ts">
import { OrganizationSwitcher } from '@clerk/vue'
</script>
<template>
<OrganizationSwitcher />
</template>
Usage with JavaScript
The following methods available on an instance of the class are used to render and control the <OrganizationSwitcher />
component:
The following examples assume that you have followed the in order to add Clerk to your JavaScript application.
mountOrganizationSwitcher()
Render the <OrganizationSwitcher />
component to an HTML <div>
element.
function mountOrganizationSwitcher(node: HTMLDivElement, props?: OrganizationSwitcherProps): void
- Name
node
- Type
HTMLDivElement
- Description
The
<div>
element used to render in the<OrganizationSwitcher />
component
- Name
props?
- Type
OrganizationSwitcherProps
- Description
The properties to pass to the
<OrganizationSwitcher />
component
import { Clerk } from '@clerk/clerk-js'
// Initialize Clerk with your Clerk Publishable Key
const clerkPubKey = import.meta.env.VITE_CLERK_PUBLISHABLE_KEY
const clerk = new Clerk(clerkPubKey)
await clerk.load()
document.getElementById('app').innerHTML = `
<div id="organization-switcher"></div>
`
const orgSwitcherDiv = document.getElementById('organization-switcher')
clerk.mountOrganizationSwitcher(orgSwitcherDiv)
unmountOrganization Switcher()
Unmount and run cleanup on an existing <OrganizationSwitcher />
component instance.
function unmountOrganizationSwitcher(node: HTMLDivElement): void
- Name
node
- Type
HTMLDivElement
- Description
The container
<div>
element with a rendered<OrganizationSwitcher />
component instance
import { Clerk } from '@clerk/clerk-js'
// Initialize Clerk with your Clerk Publishable Key
const clerkPubKey = import.meta.env.VITE_CLERK_PUBLISHABLE_KEY
const clerk = new Clerk(clerkPubKey)
await clerk.load()
document.getElementById('app').innerHTML = `
<div id="organization-switcher"></div>
`
const orgSwitcherDiv = document.getElementById('organization-switcher')
clerk.mountOrganizationSwitcher(orgSwitcherDiv)
// ...
clerk.unmountOrganizationSwitcher(orgSwitcherDiv)
Customization
To learn about how to customize Clerk components, see the customization documentation.
Feedback
Last updated on