Social connections (OAuth)
Clerk makes it easy to add social connection capabilities to your application. With social connections, users gain frictionless access to your application by using their existing credentials from an OAuth provider (Google, Facebook, X/Twitter, etc.) without having to go through complicated registration flows. Social connections are designed to simplify the sign-up and sign-in process for the end-user, resulting in higher conversion rates, a streamlined user data collection flow, and an overall better user experience.
The easiest way to add social connections is by using Clerk's prebuilt components. If you prefer a more custom solution, check out how to build a completely custom social connection flow.
Before you start
- You need to create a Clerk Application in your Clerk Dashboard. For more information, check out the Set up your application guide.
- You need to install the correct SDK for your application. You can find steps on how to do so through Clerk's quickstart guides.
Configuration
For development instances, Clerk uses pre-configured shared OAuth credentials and redirect URIs to make the development flow as smooth as possible. This means that you can enable most social providers without additional configuration.
To enable a social connection:
- Navigate to the Clerk Dashboard and select your application.
- In the navigation sidebar, select User & Authentication > Social Connections.
- Toggle on the providers that you want to use.
For production instances, you will need to configure the provider with your OAuth credentials. Don't worry, Clerk provides dedicated guides on how to do this for each provider.
Configure additional OAuth scopes
For each provider, there is a set of OAuth scopes that are absolutely necessary for authentication to work properly with Clerk. These are pre-configured by Clerk, so you don't have to worry about them.
On top of them, you can specify any additional scopes supported by the provider. These scopes can be used to access additional user data from the provider, such as email, profile information, or other data.
To add additional OAuth scopes:
- Navigate to the Clerk Dashboard and select your application.
- In the navigation sidebar, select User & Authentication > Social Connections.
- Next to the provider you want to configure, select the settings icon.
- Under Use custom credentials, you can add additional OAuth scopes in the Scopes field.
Request additional OAuth scopes after sign-up
With Clerk, you can request additional OAuth scopes even after a user has signed up.
For Clerk Components, this is handled automatically by Clerk. You only have to pass the additionalOAuthScopes
prop in the <UserProfile/>
component or the corresponding userProfileProp
in the <UserButton />
component, with any additional OAuth scope you would like per provider.
Get an OAuth access token for a social sign-in provider
You can use a provider's OAuth access token to access user data from that provider in addition to their data from Clerk.
To retrieve the OAuth access token for a user, use the getUserOauthAccessToken()
method from the JavaScript Backend SDK. Note that this method must be used in a server environment, and cannot be run on the client.
Clerk ensures that the OAuth Access Token will be always fresh so that you don't have to worry about OAuth Refresh Tokens anymore.
The following example demonstrates how to retrieve the OAuth access token for a user and use it to fetch user data from the Notion API. It assumes:
- You have already enabled the Notion OAuth connection in the Clerk Dashboard.
- The user has already connected their Notion account to your application.
- The user has the correct permissions to access the Notion API.
This example is written for Next.js App Router, but is supported by any React meta framework, such as Remix or Gatsby.
import { auth, clerkClient } from "@clerk/nextjs/server";
import { NextResponse } from "next/server";
export async function GET() {
const { userId } = auth();
if (!userId) {
return NextResponse.json({ message: "User not found" });
}
// Get the OAuth access token for the user
const provider = "oauth_notion";
const clerkResponse = await clerkClient().users.getUserOauthAccessToken(
userId,
provider
);
const accessToken = clerkResponse[0].token;
// Fetch the user data from the Notion API
// This endpoint fetches a list of users
// https://developers.notion.com/reference/get-users
const notionUrl = "https://api.notion.com/v1/users";
const notionResponse = await fetch(notionUrl, {
headers: {
Authorization: `Bearer ${accessToken}`,
"Notion-Version": "2022-06-28",
},
});
// Handle the response from the Notion API
const notionData = await notionResponse.json();
return NextResponse.json({ message: notionData });
}
Add social connection after sign-up
For each OAuth provider, you can disable the option to sign up and sign in to your application using the provider. This is especially useful for users that want to connect their OAuth account after authentication.
For example, say your application wants to read a user's GitHub repository data but doesn't want to allow the user to authenticate with their GitHub account. The user can sign up with their email and password, or whatever method you choose, and then afterwards, connect their GitHub account to your application.
After sign-up, connections can be made through our <UserProfile/>
component, or with a custom flow.
To disable the option for users to sign up and sign in with a provider:
- Navigate to the Clerk Dashboard.
- In the navigation sidebar, select User & Authentication > Social Connections.
- Next to the OAuth provider, select the settings icon.
- Toggle off Enable for sign-up and sign-in.
Connecting to OAuth providers while signed in
When signed in, a user can connect to further OAuth providers as well. There is no need to perform another sign-up.
When using Clerk's Account Portal pages, users can see which providers they have already connected to and which ones they can still connect to on their User Profile page.
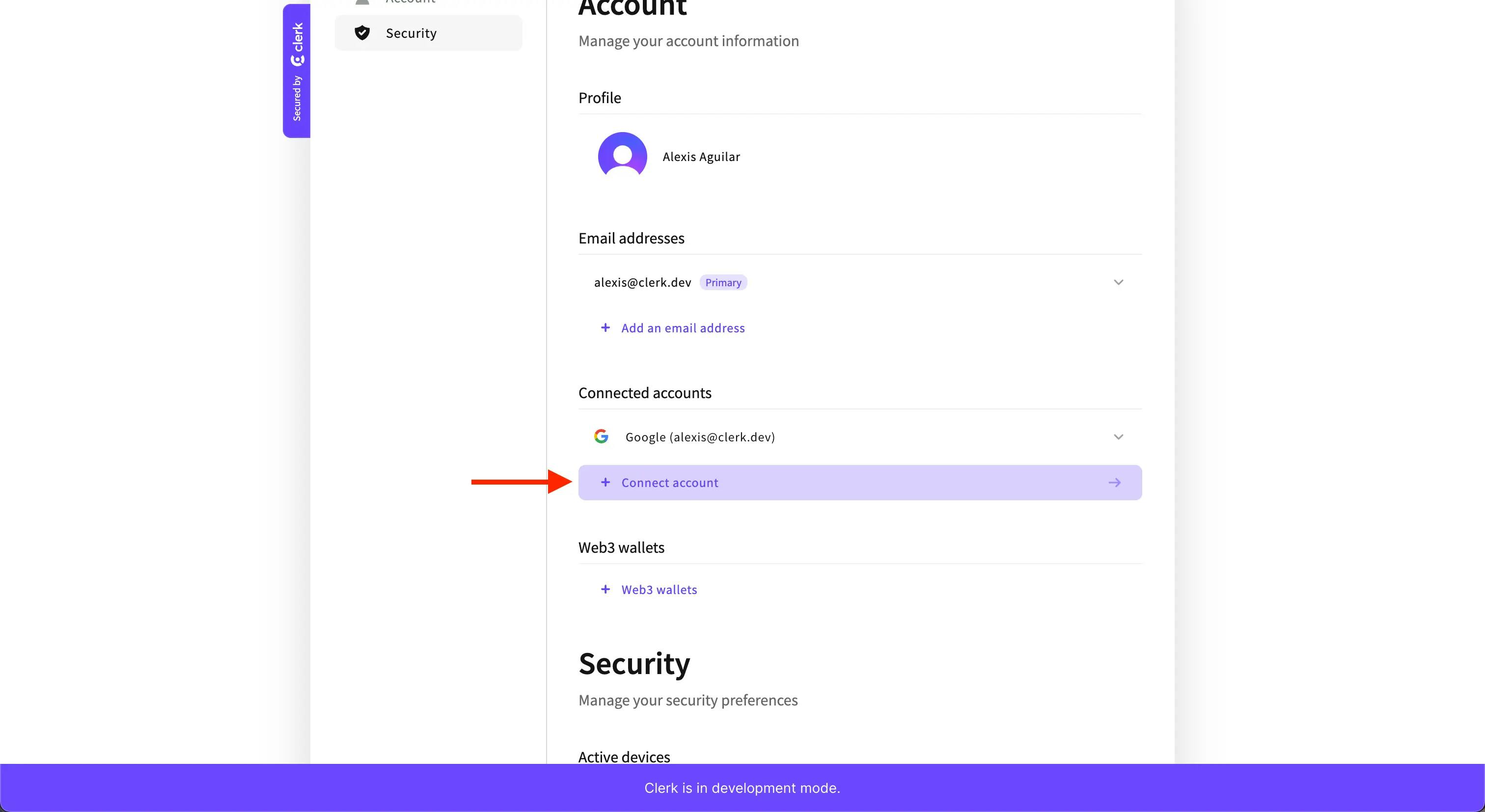
If you are using Clerk's prebuilt components, you can use the <UserProfile/>
component to allow users to connect to further OAuth providers.
OAuth for Expo applications
With Clerk, you can add OAuth flows in your Expo applications.
Clerk ensures that security critical nonces will be passed only to allowlisted URLs when the OAuth flow is complete in native browsers or webviews.
So for maximum security in your production instances, you need to allowlist your custom redirect URLs via the Clerk Dashboard or the Clerk Backend API.
To allowlist a redirect URL via the Clerk Dashboard:
- Navigate to the Clerk Dashboard.
- In the navigation sidebar, select User & Authentication > Social Connections.
- Scroll to the Allowlist for mobile OAuth redirect section and add your redirect URLs.